Create Bank Connection
Sandbox Environment Access
Our Sandbox environment allows you to simulate users and financial institutions with the full Dapi API workflow.
For more details check Get Started with Sandbox.
Bank Connection
Dapi Bank Connection
is a tokenized access to one bank account. The Bank Connection parameters have to be used during all API calls.
A Bank Connection
represents a user's connection to one bank. If the same user connects 2 banks on your application, there will be also 2 separate Bank Connection objects.
Bank Connection Using Connect Layer
All Bank Connections need to be established through Dapi's Connect Layer. Connect Layer is already embedded in the SDK and allows the users to choose the desired bank and provide their credentials.
var ba = null;
var handler = Dapi.create({
environment: Dapi.environments.sandbox, //or .production
//
// removed for examples purposes.
//
onReady: function() {
handler.open(); // opens the Connect widget
},
});
As mentioned in previous examples handler.open()
invokes the opening of the Connect Layer widget.
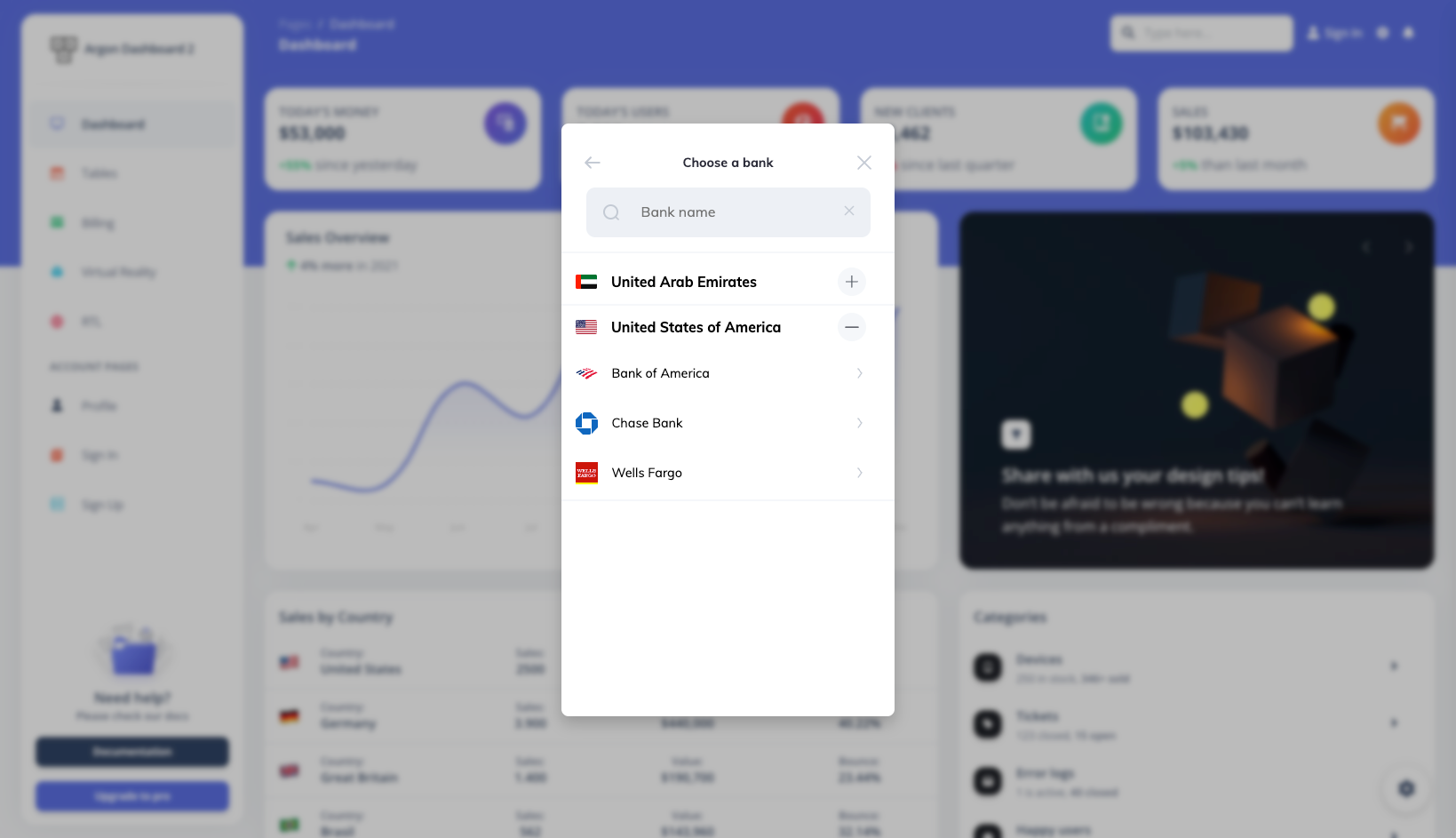
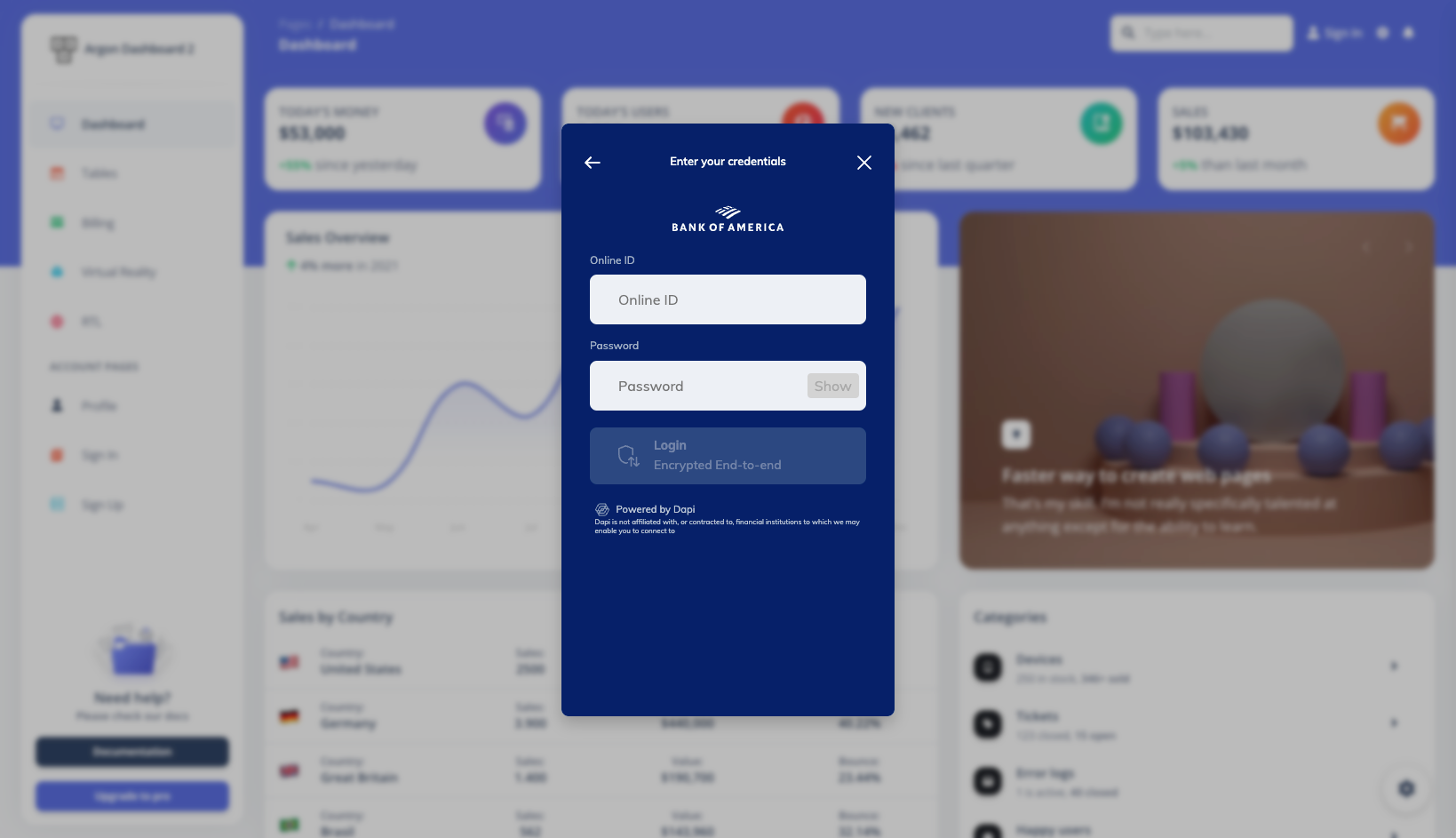
You should listen to the onSuccessfulLogin
and onFailedLogin
callbacks in order to know whether the login succeeds or fails.
After a successful login, you get a bankAccount
object, which includes all the necessary information for any consequent API calls:
- Accessing the Data API endpoints:
ba.data
- Accessing the Payment API endpoints:
ba.payment
- etc.
Example for calling the Data API getIdentity endpoint after a successful bank connection:
onSuccessfulLogin: function(bankAccount) {
ba = bankAccount;
ba.data.getIdentity()
.then((identityResponse)=>{
if (identityResponse.status === "done") {
console.log(identityResponse.identity)
} else {
console.error("API Responded with an error")
console.dir(identityResponse)
}
})
},
onFailedLogin: function(err) {
if (err != null) {
console.log("Error");
console.log(err);
} else {
console.log("No error");
}
},
Bank Connection From Cache or Database
Since the Web SDK supports local caching, you can get access to all the connected accounts on a particular clientUserID
.
Note
The
clientUserID
needs to be unique per user on your app. Otherwise, you will get access to all the bank accounts connected in that browser.
const bankAccounts = Dapi.getCachedBankAccounts("CLIENT_USER_ID")
const bankAccountParams = bankAccounts.map(bankAccount => bankAccount.flush())
// You can save this bankAccountParams on your server side
After you got access to these bankAccounts
, you can optionally save them on your server for later use. For future initialization, you can get these from your server and initialize them one by one again.
//get bankAccountParams from your server
//initialize DapiBankAccount based on a bankAccountParam
var dapiBA = new DapiBankAccount(bankAccountParams[0])
You can then call the data and payment endpoints on the dapiBA
variable.
All set!
That's it. You can use Bank Connection to collect data or initiate payments!
Account Metadata
A method for obtaining information about the user's bank. Useful for knowing special capabilities or restrictions for the specific financial institution.
Parameters
Parameter | Type | Description |
---|---|---|
clientHeaders | Object | More details |
clientBody | Object | More details |
Response
Check the Meta Data Response
Example
ba.metadata.getAccounts()
.then(metadataAccountsResponse => {
if(metadataAccountsResponse.status === "done") {
console.dir(metadataAccountsResponse)
} else {
console.error("API Responded with an error")
console.dir(metadataAccountsResponse)
}
})
.catch(error => {
console.dir(error)
})
Updated about 1 year ago