Data API
Data APIs provide all the functionality required to obtain information about your users and their financial data.
The Data APIs are:
- Identity - Provides information about the user
- Bank Accounts - Provides information about all the sub-accounts that the user has in their bank account.
- Account Transactions - Provides a complete list of transactions that the user has performed from a specific bank account
- Bank Cards - Provides information about the credit cards of the user
- Cards Transactions - Provides a complete list of transactions that the user has performed from a specific bank card
Identity
Get the identity information related to your user.
Parameters
Parameter | Description |
---|---|
bankConnection | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
Response
Check the Identity Response
Example
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
Dapi.shared.identity(bankConnection: connection) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiMetaData)
print(error.dapiErrorMessage)
}
}
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
[Dapi.shared identityWithBankConnection:connection
completion:^(DAPIIdentityResponse * _Nullable response , NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@",response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Bank Accounts
Provides information about all the sub-accounts that the user has in their bank account.
Parameters
Parameter | Description |
---|---|
bankConnection | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
Response
Check the Bank Accounts Response
Example
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
Dapi.shared.bankAccounts(bankConnection: connection) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiErrorMessage)
print(error.dapiMetaData)
}
}
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
[Dapi.shared bankAccountsWithBankConnection:connection
completion:^(DAPIBankAccountsResponse * _Nullable response,
NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@",response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Present Account Selection
Open Dapi's Accounts Screen to allow your user to select an account and get the result via closures accountDidSelect
and accountSelectionFailed
.
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
let bankAccountsVC = DAPIBankAccountsVC(bankConnection: connection)
bankAccountsVC.accountDidSelect = { account in
print(account)
}
bankAccountsVC.accountSelectionFailed = { error in
print(error)
}
self.present(bankAccountsVC, animated: true)
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
DAPIBankAccountsVC *accountVC = [[DAPIBankAccountsVC alloc] initWithBankConnection: connection
[accountVC setAccountDidSelect:^(DAPIBankAccount * _Nonnull account) {
NSLog(@"[DAPI] Success: %@",account.name);
}];
[accountVC setAccountSelectionFailed:^(NSError * _Nullable error) {
NSLog(@"[DAPI] error: %@",error.dapiErrorMessage);
}];
[self presentViewController:accountVC animated:YES completion:nil];
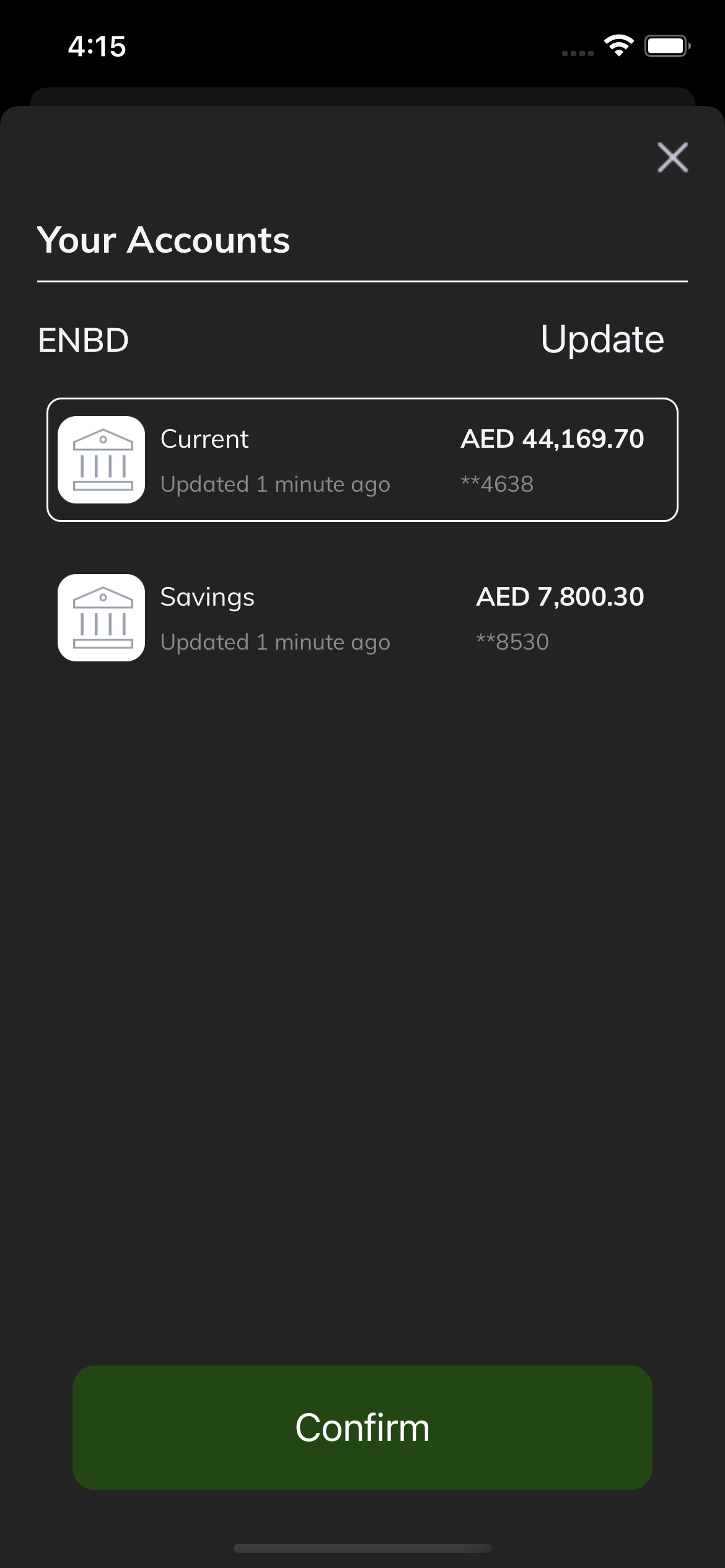
Bank Cards
A method for obtaining and displaying credit cards of the user.
Parameters
Parameter | Description |
---|---|
bankConnection | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
Response
Check the Bank Cards Response
Example
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
Dapi.shared.bankCards(bankConnection: connection) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiErrorMessage)
print(error.dapiMetaData)
}
}
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
[Dapi.shared bankCardsWithBankConnection: connection
completion:^(DAPIBankCardsResponse * _Nullable response,
NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@", response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Account Transactions
A method for obtaining and displaying transactions created from user's bank accounts. The list will not be filtered. In other words, this will display all the transactions performed by the user from the specified account (not filtered by the app).
Parameters
Parameter | Description |
---|---|
bankConnection | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
bankAccountID | The ID for bank account which the transaction was performed from |
type | Enum to specify transactions type, if you didn't set it would be .default value. default → Each transaction won't include category and brandDetails enriched → Each transaction object will have category and brandDetails properties. categorized → Each transaction object will have a category property. |
startDate | Start date of transactions history range, Must be in the following format yyyy-MM-dd |
endDate | End date of transactions history range, Must be in the following format yyyy-MM-dd |
Response
Check the Account Transactions Response
Example
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy-MM-dd"
Dapi.shared.accountTransactions(bankConnection: connection,
bankAccountID: <##bank_account_ID##>,
type: .enriched,
from: dateFormatter.date(from: "2021-01-25"),
to: dateFormatter.date(from: "2021-04-25")) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiErrorMessage)
print(error.dapiMetaData)
}
}
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"yyyy-MM-dd"];
[Dapi.shared accountTransactionsWithBankConnection: connection
bankAccountID: @"<##bank_account_ID##>"
type:DAPITransactionTypeDefault
from:[dateFormatter dateFromString: @"2022-01-01"]
to:[dateFormatter dateFromString: @"2022-02-09"]
completion:^(DAPITransactionsResponse * _Nullable response, NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@",response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Cards Transactions
A method for obtaining and displaying transactions created from a card. The list will not be filtered. In other words, this will display all the transactions performed by the user from the specified credit card (not filtered by the app).
Parameters
Parameter | Description |
---|---|
bankConnection | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
cardID | The ID for card which the transaction was performed from |
type | Enum to specify transactions type, if you didn't set it would be .default value. default → Each transaction won't include category and brandDetails. enriched → Each transaction object will have category and brandDetails properties. categorized → Each transaction object will have a category property. |
startDate | Start date of transactions history range, Must be in the following format yyyy-MM-dd |
endDate | End date of transactions history range, Must be in the following format yyyy-MM-dd |
Response
Check the Cards Transactions Response
Example
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy-MM-dd"
Dapi.shared.cardTransactions(bankConnection: connection,
cardID: <##card_ID##>,
type: .enriched,
from: dateFormatter.date(from: "2021-01-25"),
to: dateFormatter.date(from: "2021-04-25")) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiErrorMessage)
print(error.dapiMetaData)
}
}
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"yyyy-MM-dd"];
[Dapi.shared cardTransactionsWithBankConnection: connection
cardID: @"<##card_ID##>>"
type:DAPITransactionTypeDefault
from:[dateFormatter dateFromString: @"2022-01-01"]
to:[dateFormatter dateFromString: @"2022-02-09"]
completion:^(DAPITransactionsResponse * _Nullable response, NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@",response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Date range of the transactions that can be retrieved varies for each bank. The range supported by the users bank is shown in the response parameter
transactionRange
ofGet Accounts Metadata
endpoint. If the date range you provide is bigger than thetransactionRange
you'll getINVALID_DATE_RANGE
error.To make sure you always send a valid date range you should check the transactionsRange.
Example:private func getTransactionsFor(numberOfMonths: Int ) { let days = numberOfMonths * 30 let daySeconds = 24*60*60 self.connection?.getAccountMetadata({ response, error, operationID in let transactionsRetentionPeriod = response?.transactionsRetentionPeriod let now = Date() var fromDate = Date() if(transactionsRetentionPeriod == nil) { //Can't check transactions range.. return } if(Int(transactionsRetentionPeriod!.magnitude) / daySeconds > days) { //numberOfMonths is within the transaction range fromDate = Calendar.current.date(byAdding: .day, value: -days, to: now)! } else { //numberOfMonths is NOT within the transaction range, so we query only for the allowed range fromDate = Calendar.current.date(byAdding: .second, value: Int(transactionsRetentionPeriod!.magnitude * -1), to: now)! } if let accs = self.connection?.accounts, !accs.isEmpty { self.connection?.getTransactionsFor(accs[0], from: fromDate, to: Date(), type: .default, completion: { (transactions, error, opID) in }) } }) }
Updated over 1 year ago