Wire API (US only)
Wire API provides all the functionality required to manage beneficiaries and initiate payments on your user's behalf.
The Payment APIs are:
- CreateWireTransfer - The easiest and most efficient way to facilitate payments.
- [Not required when using CreateWireTransfer] Get Wire beneficiaries - Retrieve beneficiaries from the bank account.
- [Not required when using CreateWireTransfer] Create Wire Beneficiary - Create a beneficiary into the bank account.
- [Not required when using CreateWireTransfer] CreateWireTransferToExistingBeneficiary - Initiate a transfer to an existing wire beneficiary only.
createWireTransfer [Recommended]
createWireTransfer
automatically takes care of all requirements for adding a beneficiary as well as initiating a transfer. We recommend using createWireTransfer
to initiate a payment.
you can send money from
an account to
an account with a specific amount
.All 3 variables are optional.
Parameters
Note
Behavior of the SDK depends on the parameters that will be set.
Parameter | Description |
---|---|
toBeneficiary | Information of the recipient beneficiary. |
fromAccount | Account from where the amount must be transferred. If you don't set a from account, SDK will simply display a popup screen for your user to pick the account from our UI. If you do provide a from object, this screen won't be displayed. |
amount | Amount to be transferred. If you don't set an amount SDK will display a screen with a numpad screen for your user to enter the amount in. |
remark | A message associated with the transfer. |
Implement DapiTransferCallback to handle a successful or a failed transfer attempt.
Dapi.transferCallback = object : DapiTransferCallback {
override fun onTransferSuccess(result: DapiTransferResult.Success) {
}
override fun onTransferFailure(result: DapiTransferResult.Error) {
}
override fun willTransferAmount(result: DapiTransferResult.PreTransfer) {
}
override fun onUiDismissed() {
}
}
Dapi.setTransferListener(new OnDapiTransferListener() {
@Override
public void willTransferAmount(double amount, @NonNull DapiAccountsResponse.DapiAccount senderAccount) {
}
@Override
public void onTransferSuccess(@NonNull DapiAccountsResponse.DapiAccount account, double amount, @Nullable String reference, @Nullable String operationID) {
}
@Override
public void onTransferFailure(@Nullable DapiAccountsResponse.DapiAccount account, @NonNull DapiError error) {
}
@Override
public void onUiDismissed() {
}
});
Example - When fromAccount
Is Not Specified
val address = LinesAddress()
address.line1 = "line1"
address.line2 = "line2"
address.line3 = "line3"
val beneficiary = DapiWireBeneficiary(
address = address,
accountNumber = "65432256",
name = "John Doe",
country = "US",
receiverType = "retail",
routingNumber = "67543534",
nickname = "JohnDoe",
receiverAccountType = "checking",
firstName = "John",
lastName = "Doe",
zipCode = "92335",
state = "Arkansas",
city = "Conway",
)
connection.createWireTransfer(
toBeneficiary = beneficiary,
amount = 100.0
)
connection.createTransfer(null, null, amount);
The SDK shows UI for the user to select an account to send money from
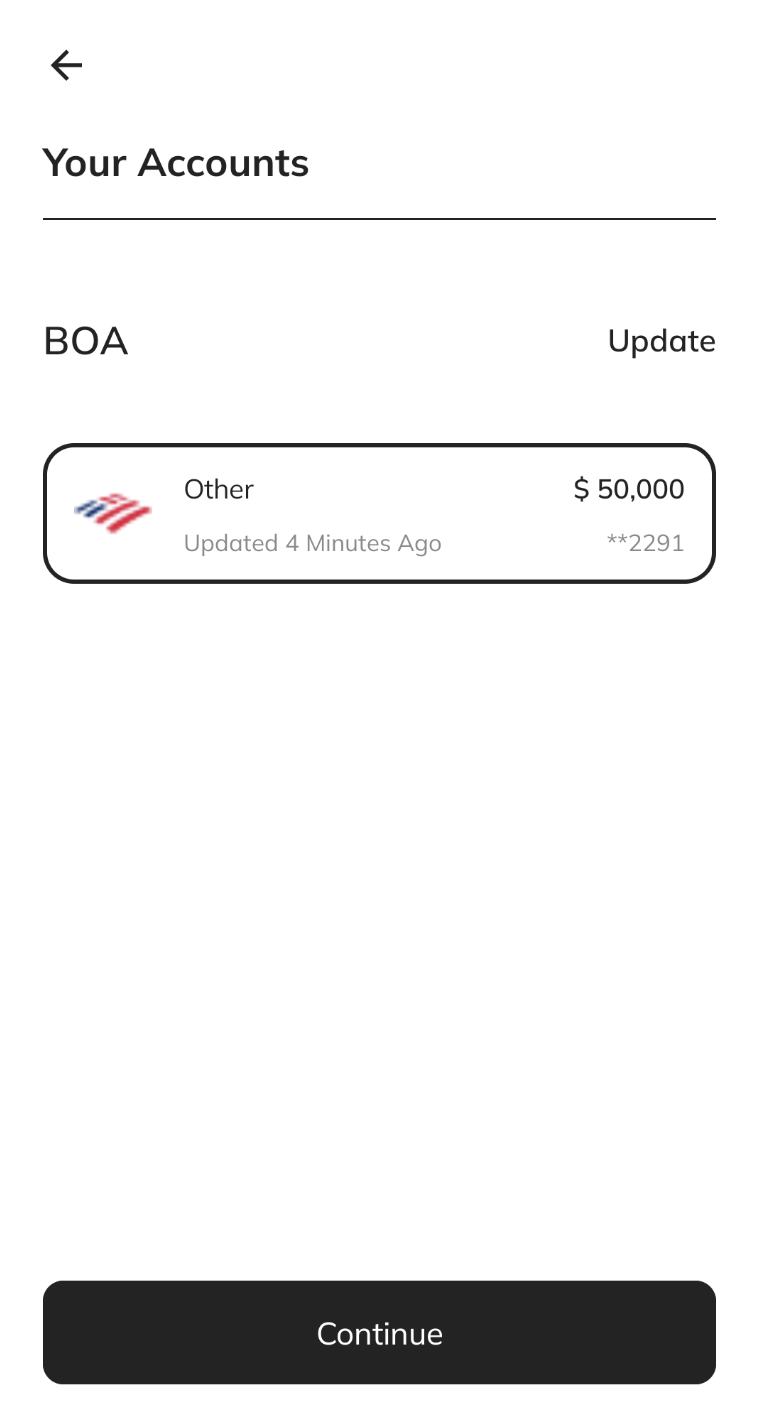
Account Selection Screen
Example - When amount
Is Not Specified
val address = LinesAddress()
address.line1 = "line1"
address.line2 = "line2"
address.line3 = "line3"
val beneficiary = DapiWireBeneficiary(
address = address,
accountNumber = "65432256",
name = "John Doe",
country = "US",
receiverType = "retail",
routingNumber = "67543534",
nickname = "JohnDoe",
receiverAccountType = "checking",
firstName = "John",
lastName = "Doe",
zipCode = "92335",
state = "Arkansas",
city = "Conway",
)
connection.createWireTransfer(
toBeneficiary = beneficiary,
fromAccount = connection.accounts?.first()
)
connection.createTransfer(connection.getAccounts().get(0));
The SDK shows UI for the user to enter the amount to send
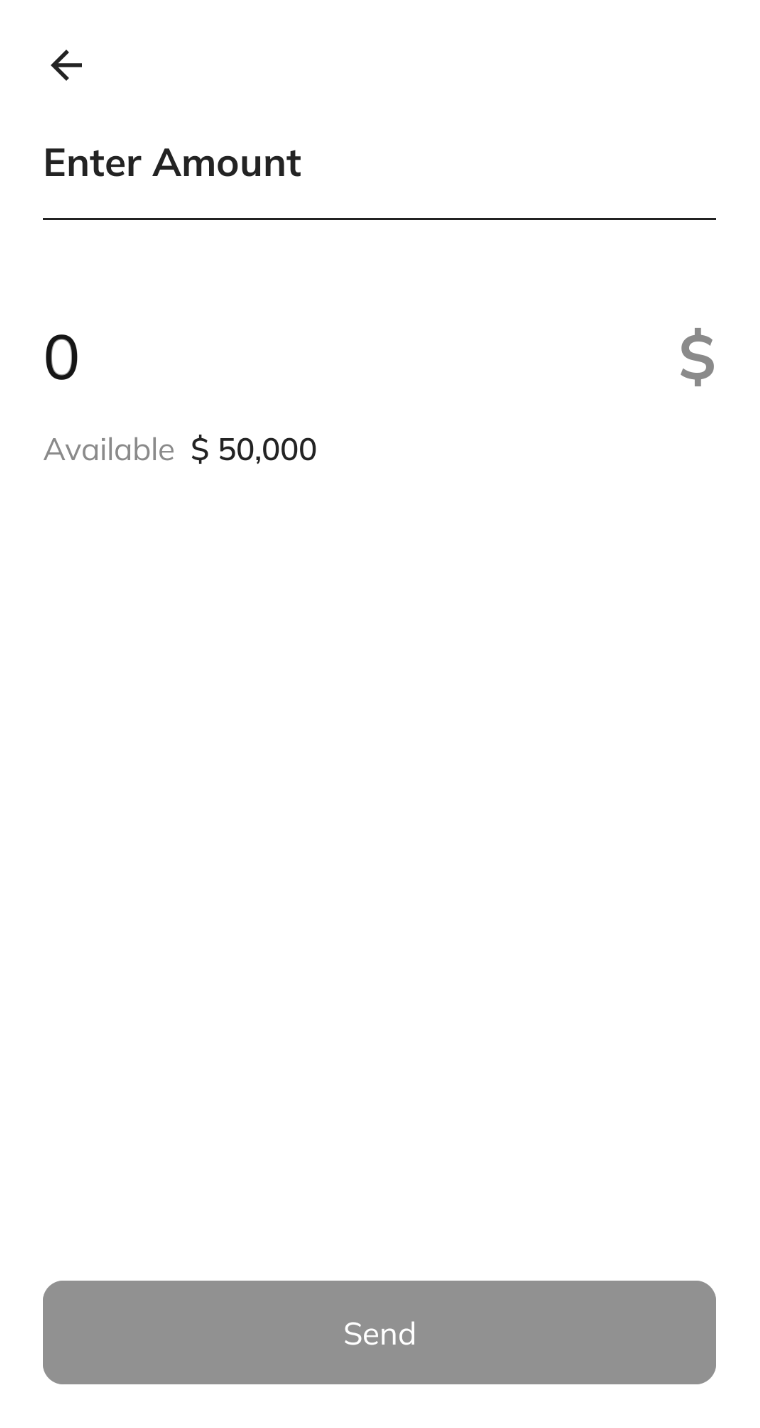
Amount Screen
Example - When amount
and fromAccount
Are Not Specified
val address = LinesAddress()
address.line1 = "line1"
address.line2 = "line2"
address.line3 = "line3"
val beneficiary = DapiWireBeneficiary(
address = address,
accountNumber = "65432256",
name = "John Doe",
country = "US",
receiverType = "retail",
routingNumber = "67543534",
nickname = "JohnDoe",
receiverAccountType = "checking",
firstName = "John",
lastName = "Doe",
zipCode = "92335",
state = "Arkansas",
city = "Conway",
)
connection.createWireTransfer(
toBeneficiary = beneficiary,
)
connection.createTransfer();
The SDK shows UI for the user to select an account to send money from then navigate to the amount screen to enter the amount to send
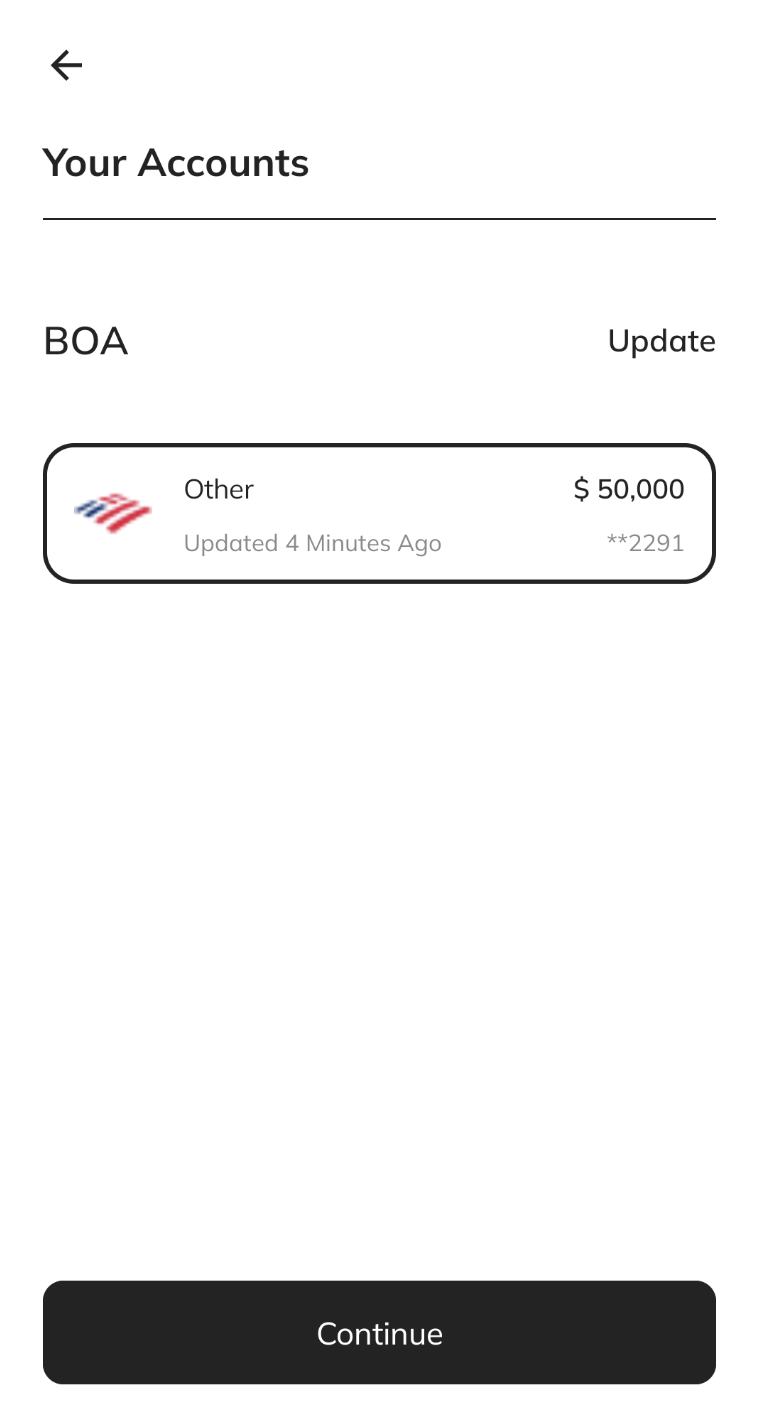
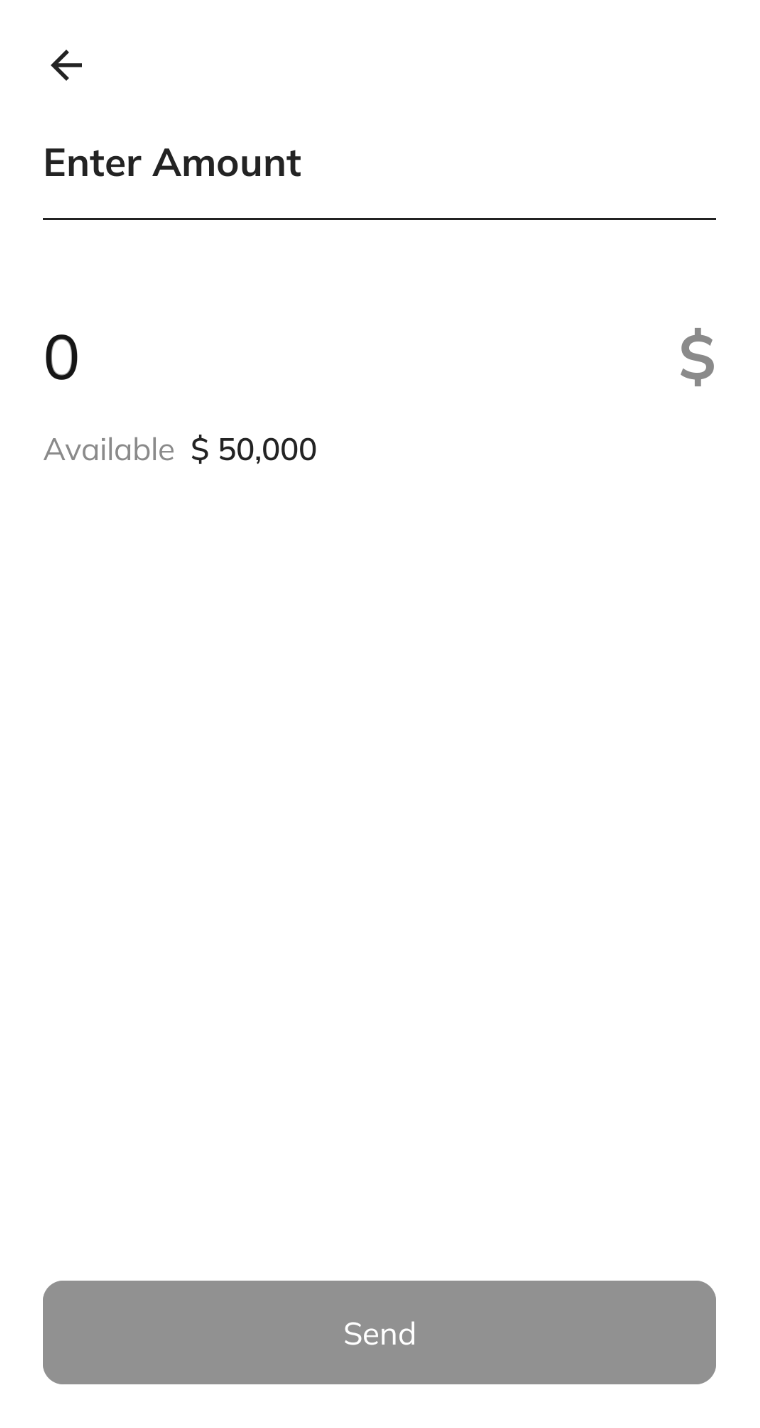
createWireTransferToExistingBeneficiary
Note
If you use
createWireTransferToExistingBeneficiary
you have to implement bank processing logic and validations on your end. You will require the use of the following APIs to process a transaction:
- Get Account
- Create Wire Beneficiary
- Get Wire Beneficiaries
createWireTransfer
abstracts all these requirements. There is no need to implement bank processing logic or validations. Dapi recommends usingcreateWireTransfer
to initiate a payment.
A method for sending money to an existing wire beneficiary.
Parameters
Parameter | Description |
---|---|
fromAccount | Account from where the amount must be transferred. |
toBeneficiaryID | The id of the wire beneficiary to which the money must be transferred. Obtain by connection.getWireBeneficiaries() |
amount | Amount to be transferred |
Example
connection.createWireTransferToExistingBeneficiary(
fromAccount = connection.accounts!!.first(),
toBeneficiaryID = wireBeneficiaryID,
amount = amount,
remark = "test"
)
connection.getBeneficiaries((beneficiariesResponse) -> {
connection.createTransferToExistingBeneficiary(
connection.getAccounts().get(0),
beneficiariesResponse.getBeneficiaries().get(0).getId(),
amount
);
return null;
}, (error) -> {
return null;
});
Beneficiary Not Activated error on Sandbox
This error occurs If you make a transfer on Sandbox to wrong or random beneficiary details, instead you should make the beneficiary details to be of another sandbox user.
To get the beneficiary details of a sandbox user you can do the following:
1- Open your app on the dashboard and navigate to the Sandbox tab. You'll see the Sandbox Users table.
2- Click an account from the Accounts column.
3- A pop up shows up containing beneficiary details.
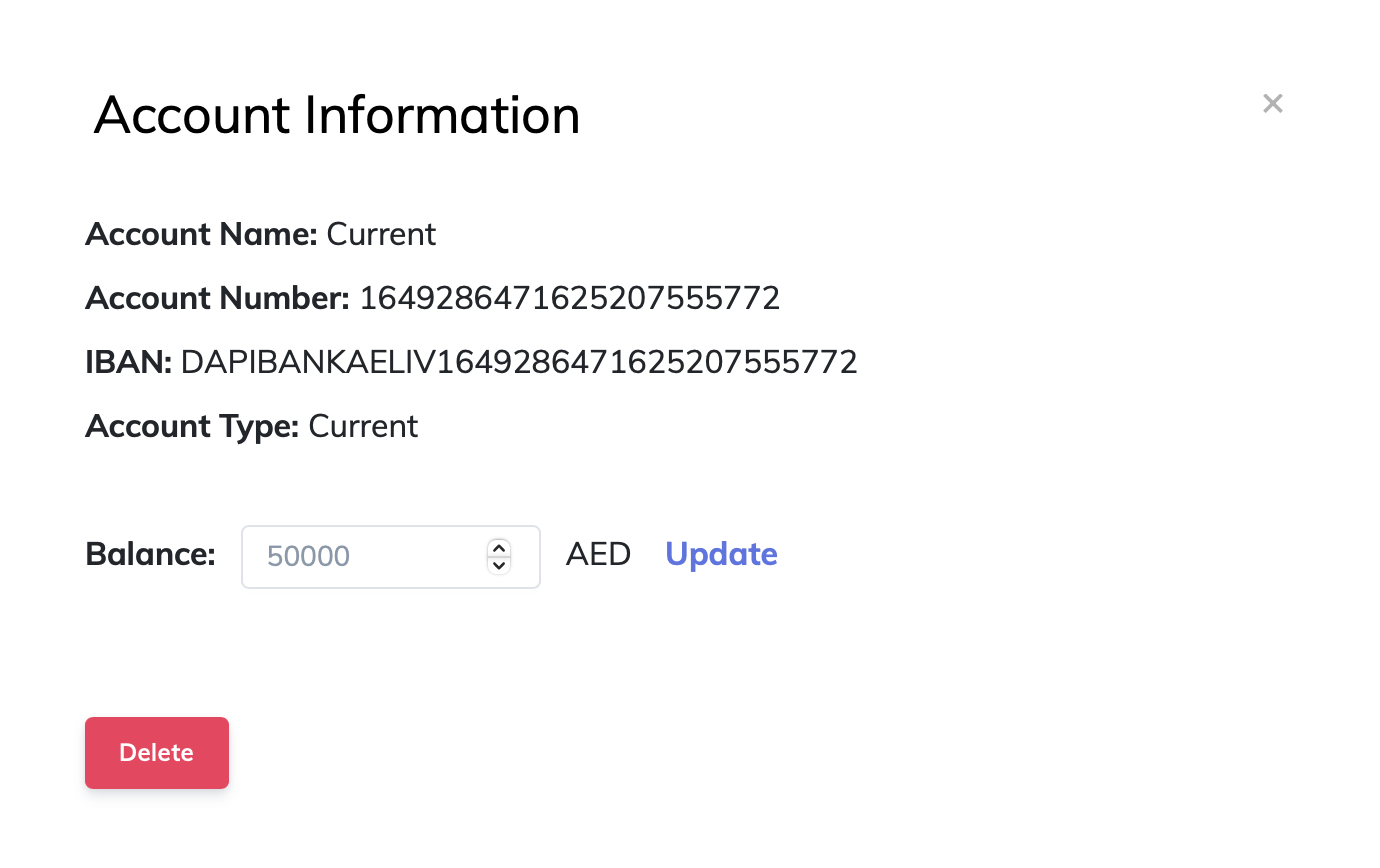
Sandbox Account Beneficiary details
createWireBeneficiary
A method for adding a new wire beneficiary
Parameters
Parameter | Description |
---|---|
beneficiary | Information of the wire beneficiary to add. |
Example
val address = LinesAddress()
address.line1 = "line1"
address.line2 = "line2"
address.line3 = "line3"
val beneficiary = DapiWireBeneficiary(
address = address,
accountNumber = "65432256",
name = "John Doe",
country = "US",
receiverType = "retail",
routingNumber = "67543534",
nickname = "JohnDoe",
receiverAccountType = "checking",
firstName = "John",
lastName = "Doe",
zipCode = "92335",
state = "Arkansas",
city = "Conway",
)
connection.createWireBeneficiary(
beneficiary = beneficiary,
onSuccess = {
},
onFailure = {
}
)
LinesAddress lineAddress = new LinesAddress();
lineAddress.setLine1("baniyas road");
lineAddress.setLine2("dubai");
lineAddress.setLine3("united arab emirates");
DapiBeneficiary beneficiary = new DapiBeneficiary(
lineAddress,
"0959040184901",
"John Doe",
"Emirates NBD Bank PJSC",
"EBILAEAD",
"AE140260000959040184901",
"UNITED ARAB EMIRATES",
"Baniyas Road Deira PO Box 777 Dubai UAE",
"Emirates NBD Bank PJSC",
"+0585859206"
);
connection.createBeneficiary(beneficiary, (createBeneficiaryResponse) -> {
return null;
}, (error) -> {
return null;
});
getWireBeneficiaries
A method for obtaining registered wire beneficiaries
Parameters
Method does not receive any parameter.
Example
connection.getWireBeneficiaries({ beneficiariesResponse ->
}, { error ->
})
connection.getBeneficiaries((beneficiariesResponse) -> {
return null;
}, (error) -> {
return null;
});
Updated 8 months ago