ACH API (US only)
Dapi AutoFlow ACH Pull Transfer
ACH Pull Transfer Auto Flow
automatically takes care of all requirements for initiating a transfer.
Using Dapi AutoFlow you can easily create an ACH Pull transfer and listen on the success or failure results
Initializer Parameters
Description | Type | Description |
---|---|---|
bankConnection | DAPIBankConnection REQUIRED | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
account | DAPIBankAccount? OPTIONAL | Account where the amount must be transferred from. If you don't provide it, AutoFlow will start display bank accounts screen for your user to pick the account from it. |
amount | Float? OPTIONAL | Amount to be transferred. If you don't set an amount SDK will display a screen with a numpad screen for your user to enter the amount in. |
description | String REQUIRED | This is the id you can use to track the transaction. |
AutoFlow Examples for Different Use Cases
Note: No need to pass nil to any OPTIONAL parameter, just don't include it in its Initializer parameter.
//#1
// autoFlowVC will start with Account selection screen then enter amount
let autoFlowVC = DAPIAutoFlowVC(bankConnectionForACHPullTransfer: connection,
description: "Transfer Description")
//#2
// autoFlowVC will start with enter amount screen, since you already pass the account.
let autoFlowVC = DAPIAutoFlowVC(bankConnectionForACHPullTransfer: connection,
account: #account#,
description: "Transfer Description")
//#3
// autoFlowVC will start with account selection screen, and enter amount screen will not be shown, since you already pass the amount.
let autoFlowVC = DAPIAutoFlowVC(bankConnection: connection,
amount: 2.0 ,
description: "Transfer Description")
// After choose the way you want to initialize autoFlowVC, presnet it.
self.present(autoFlowVC, animated: true)
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
DAPIAutoFlowVC *autoflow = [[DAPIAutoFlowVC alloc] initWithBankConnectionForACHPullTransfer:[self selectedConnection]
account:account
amount:100
description:@"description"];
[self presentViewController:autoflow animated:YES completion:nil];
ACHPull AutoFlow Result Handling
You can handle transfer results using delegate autoFlowDelegate
or closures transferDidFail
and transferDidSucceed
let autoFlowVC = DAPIAutoFlowVC(bankConnectionForACHPullTransfer: connection,
description: "Transfer Description")
//closures fired when transfer failed
autoFlowVC.transferDidFail = { error in
print(error.dapiMetaData)
print(error.dapiErrorMessage)
}
//closures fired when transfer succeeded
autoFlowVC.transferDidSucceed = { transfer in
print(transfer.operationID)
print(transfer.referenceNumber)
print(transfer.remark)
}
self.present(autoFlowVC, animated: true)
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
DAPIAutoFlowVC *autoflow = [[DAPIAutoFlowVC alloc] initWithBankConnectionForACHPullTransfer:[self selectedConnection]
account:account
amount:100
description:@"description"];
[autoflow setTransferDidSucceed:^(DAPITransferResult * _Nonnull data) {
NSLog(@"[DAPI] Success: data %@", data.dictionaryRepresentation);
}];
[autoflow setTransferDidFail:^(NSError * _Nonnull error ) {
NSLog(@"[DAPI] error: data %@",error.dapiOperationID);
NSLog(@"[DAPI] type: data %@",error.dapiErrorType);
NSLog(@"[DAPI] code: data %@",error.dapiStatusCode);
NSLog(@"[DAPI] code: data %@",error.dictionaryRepresentation);
NSDictionary *coolDownPeriod = [error.dictionaryRepresentation objectForKey:@"coolDownPeriod"];
NSNumber *value = [coolDownPeriod objectForKey:@"value"];
NSString *unit = [coolDownPeriod objectForKey:@"unit"];
}];
[self presentViewController:autoflow animated:YES completion:nil];
Complete Example using autoFlowDelegate
autoFlowDelegate
let autoFlowVC = DAPIAutoFlowVC(bankConnectionForACHPullTransfer: connection,
description: "Transfer Description")
autoFlowVC.autoFlowDelegate = self
self.present(autoFlowVC, animated: true)
--------------------------------------------------------------------------------------
extension ViewController: DAPIAutoFlowDelegate {
func autoFlow(_ autoFlow: DAPIAutoFlowVC,
willTransferAmount amount: Float,
fromAccount senderAccount: DAPIBankAccount) {
print(#function)
}
func autoFlow(_ autoFlow: DAPIAutoFlowVC,
achPullTransferDidFailFrom senderAccount: DAPIBankAccount?,
with error: Error) {
print(#function)
}
func autoFlow(_ autoFlow: DAPIAutoFlowVC,
transferDidSuccessFrom senderAccount: DAPIBankAccount,
transferResult: DAPITransferResult) {
print(transferResult.referenceNumber)
print(transferResult.remark)
print(transferResult.operationID)
}
func autoFlowUserDidCanceled(_ autoFlow: DAPIAutoFlowVC) {
print(#function)
}
}
Complete Visual Example
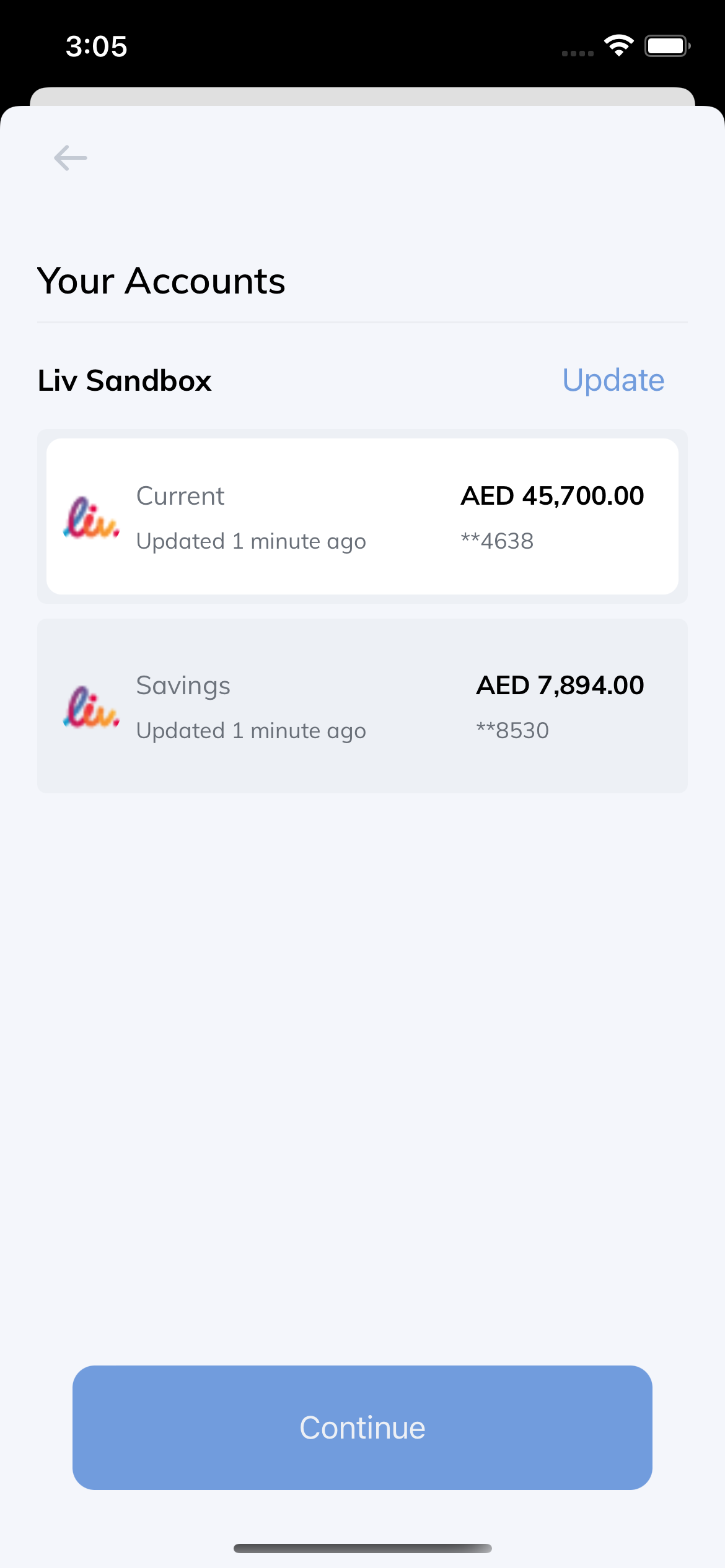
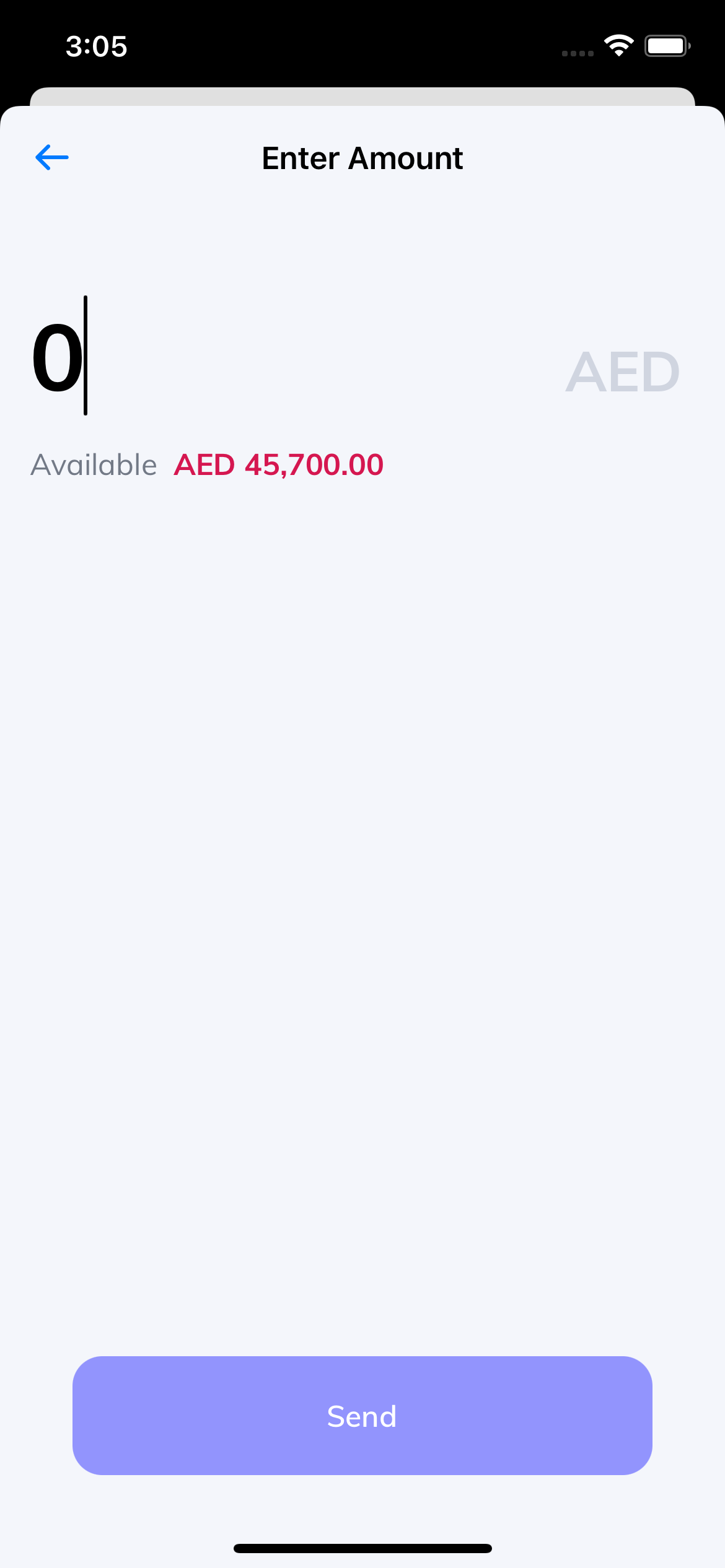
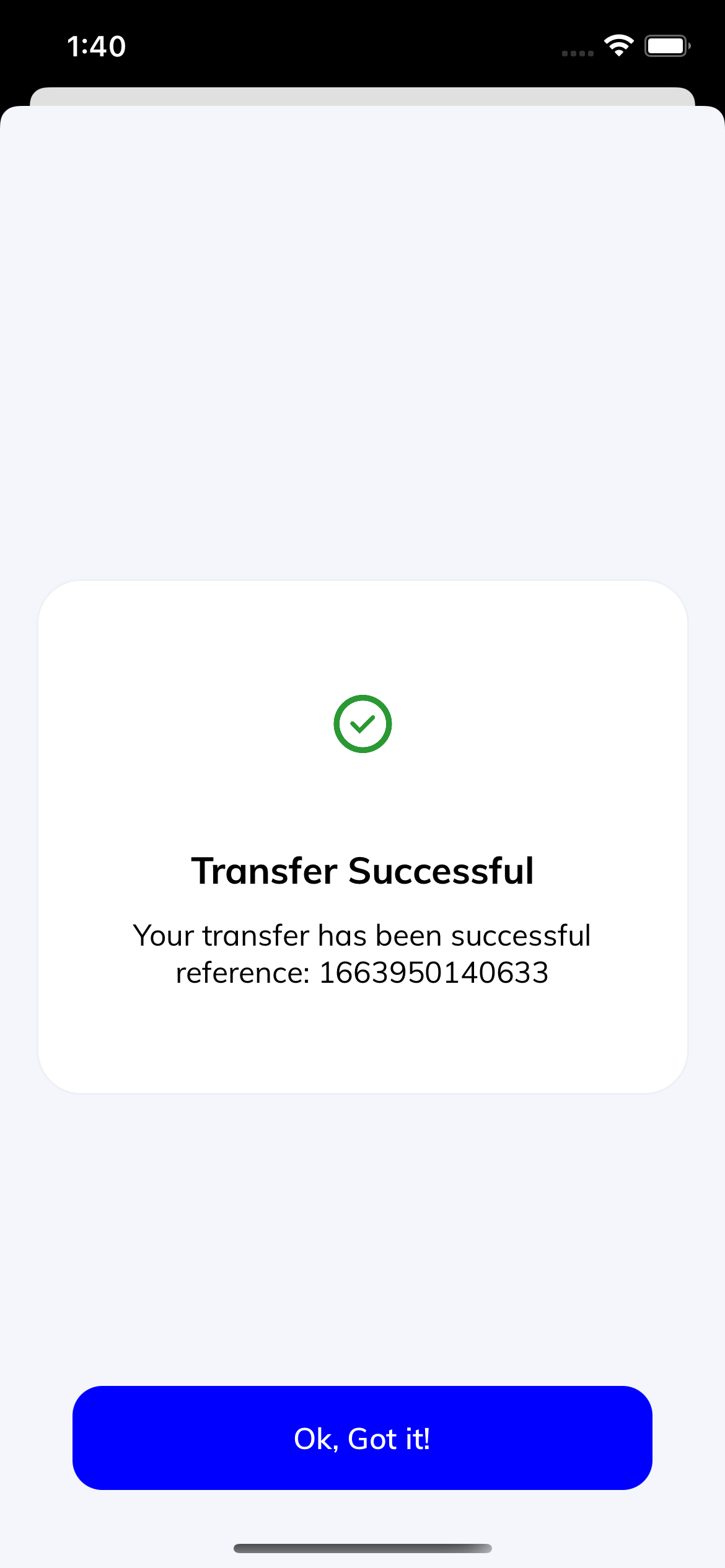
AutoFlow Transfer Without UI
Use this approach only if you don't want to use any pre-built UI elements from the SDK.
Parameters
Description | Type | Description |
---|---|---|
bankConnection | DAPIBankConnection REQUIRED | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
senderBankAccount | DAPIBankAccount REQUIRED | Account where the amount must be transferred from. |
amount | Float REQUIRED | Amount to be transferred. |
description | String REQUIRED | This is the id you can use to track the transaction. |
Response
Check Create ACH Pull Transfer Response
Example
Dapi.shared.createACHPullTransfer(bankConnection: connection,
senderBankAccount: #sender_account#,
amount: 20,
description: "Transfer Description") { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiErrorMessage)
}
}
[Dapi.shared createACHPullTransferWithBankConnection:[self selectedConnection]
senderBankAccountId:@"accountID"
amount:100
description:@"description"
completion:^(DAPITransferResult * _Nullable response, NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@",response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Updated 5 months ago