Data API
Data APIs provide all the functionality required to obtain information about your users and their financial data.
The Data APIs are:
- Identity - Provides information about the user
- Bank Accounts - Provides information about all the sub-accounts that the user has in their bank account.
- Account Transactions - Provides a complete list of transactions that the user has performed from a specific bank account
- Credit Cards - Provides information about the credit cards of the user
- Cards Transactions - Provides a complete list of transactions that the user has performed from a specific bank card
Identity
Parameter | Type | Description |
---|---|---|
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
ba.data.getIdentity()
.then(identityResponse => {
if(identityResponse.status === "done") {
console.dir(identityResponse)
} else {
console.error("API Responded with an error")
console.dir(identityResponse)
}
})
.catch(error => {
console.dir(error)
})
Accounts
Access the sub-accounts of a bank account and balance for corresponding accounts.
Parameter | Type | Description |
---|---|---|
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
Example
ba.data.getAccounts()
.then(accountsResponse => {
if(accountsResponse.status === "done") {
console.dir(accountsResponse)
} else {
console.error("API Responded with an error")
console.dir(accountsResponse)
}
})
.catch(error => {
console.dir(error)
})
Accounts Modal
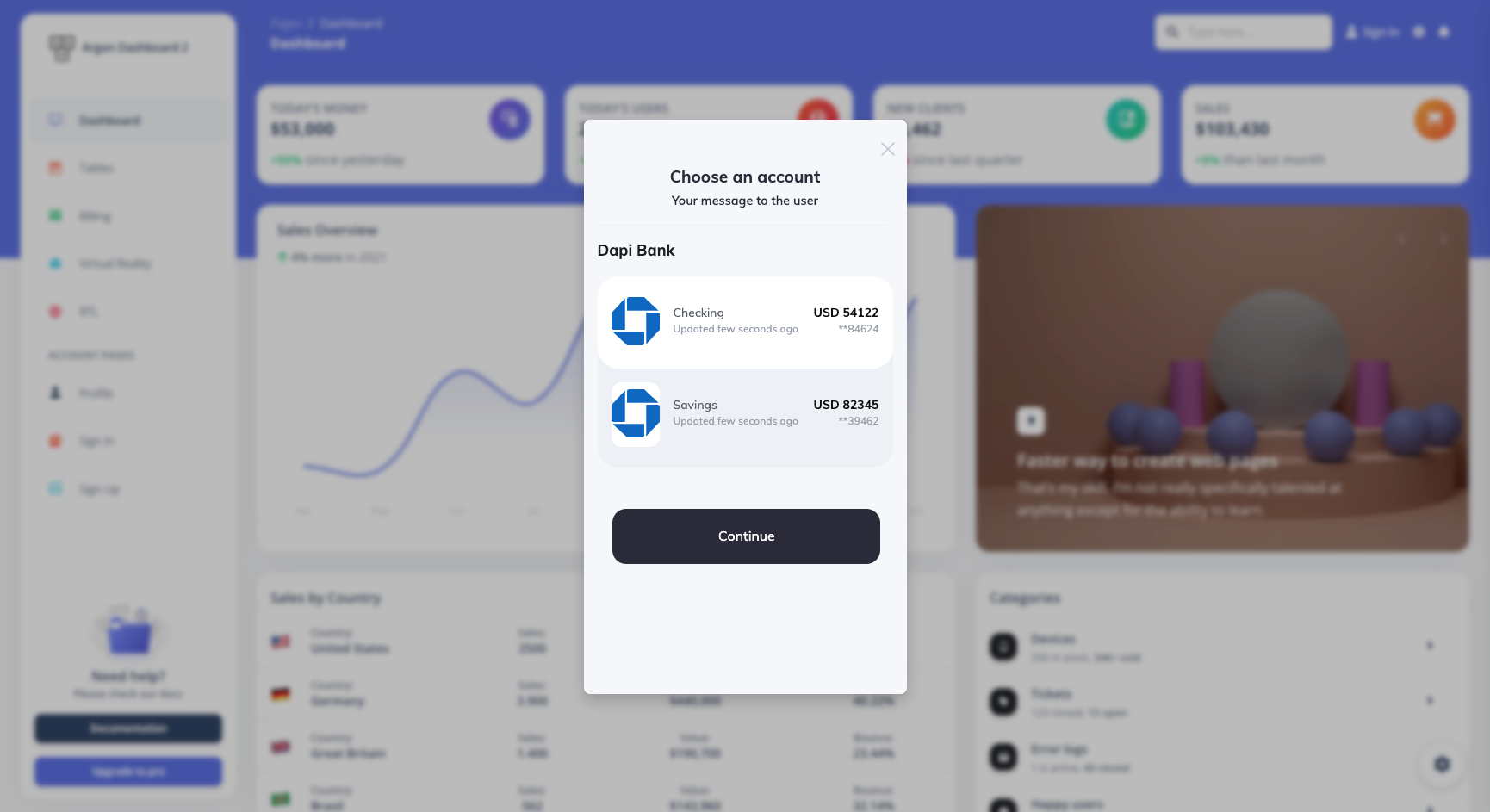
The Accounts Modal allows you to prompt the user with a screen showing all sub-accounts. The user will need to select one of the accounts in order to proceed. You can add a custom message to the user that will be displayed on the modal, such as "Select an account where you receive your salary"
or "Select an account to proceed with transfer"
Accounts Modal Complete Example
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title></title>
<meta name="author" content="" />
<meta name="description" content="" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body>
<div
style="display: flex; flex-direction: column; flex: 1; justify-content: center; align-items: center; width: 100%; height: 100%">
<h1>Client Website!!</h1>
<button style="height: 2rem;width: 20rem;background: beige;border: 1px solid black; margin-top: 2rem;"
onclick="clickMe()">
Quick Transfer
</button>
</div>
<script src="https://cdn.dapi.co/dapi/v2/sdk.js"></script>
<script>
let connectLoading = true
var ba = null
var dapi = Dapi.create({
environment: Dapi.environments.sandbox,
appKey: "YOUR_APP_KEY",
countries: ["AE"],
bundleID: "YOUR_BUNDLE_ID",
clientUserID: "CLIENT_USER_ID",
isCachedEnabled: true,
isExperimental: false,
clientHeaders: {},
clientBody: {},
onSuccessfulLogin: function (bankAccount) {
ba = bankAccount;
ba.data.getAccounts()
.then(payload => {
ba.showAccountsModal(
"Your message to the user",
payload.accounts,
(account) => {
console.dir(account)
},
() => {
console.dir("User Cancelled")
})
})
},
onFailedLogin: function (err) {
if (err != null) {
console.log("Error");
console.log(err);
} else {
console.log("No error");
}
},
onReady: function () {
connectLoading = false
},
onExit: function() {
console.log("User exited the flow")
}
});
var clickMe = function () {
if (!connectLoading) {
dapi.open();
} else {
console.error("Widget is loading. Please wait!")
}
};
</script>
</body>
</html>
Credit Cards
Access the credit cards associated with this bank account
Parameter | Type | Description |
---|---|---|
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
Example
ba.data.getCards()
.then(cardsResponse => {
if(cardsResponse.status === "done") {
console.dir(cardsResponse)
} else {
console.error("API Responded with an error")
console.dir(cardsResponse)
}
})
.catch(error => {
console.dir(error)
})
Account Transactions
Access the transactions of a given sub-account.
Parameter | Type | Description |
---|---|---|
accountID | String | The id of a particular sub account. Note: You can get this from calling getAccounts function |
fromDate | String | The start date of the transactions you want to get. It needs to be in the YYYY-MM-DD formatNB! Check the available time ranges from the Metadata |
toDate | String | The end date of the transactions you want to get. It needs to be in the YYYY-MM-DD format |
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
Example
ba.data.getTransactions(accountID, "2020-01-01", "2020-02-01")
.then(transactionsResponse => {
if(transactionsResponse.status === "done") {
console.dir(transactionsResponse)
} else {
console.error("API Responded with an error")
console.dir(transactionsResponse)
}
})
.catch(error => {
console.dir(error)
})
Get Account Transactions Response
Account Enhanced Transactions
Access the enhanced transactions of a given sub-account.
Parameter | Type | Description |
---|---|---|
accountID | String | The id of a particular sub account. Note: You can get this from calling getAccounts function |
fromDate | String | The start date of the transactions you want to get. It needs to be in the YYYY-MM-DD formatNB! Check the available time ranges from the Metadata |
toDate | String | The end date of the transactions you want to get. It needs to be in the YYYY-MM-DD format |
type | String | Specify transactions type.enriched -> Each transaction object will have category and brandDetails properties.categorized -> Each transaction object will have category property.default -> category and brandDetails won't be included in the response. |
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
ba.data.getEnhancedTransactions(accountID, "2020-01-01", "2020-02-01", "enriched")
.then(transactionsResponse => {
if(transactionsResponse.status === "done") {
console.dir(transactionsResponse)
} else {
console.error("API Responded with an error")
console.dir(transactionsResponse)
}
})
.catch(error => {
console.dir(error)
})
Credit Card Transactions
Access the transactions of a given credit card.
Parameter | Type | Description |
---|---|---|
cardID | String | The id of a particular card Note: You can get this from calling getCards function |
fromDate | String | The start date of the transactions you want to get. It needs to be in the YYYY-MM-DD format |
toDate | String | The end date of the transactions you want to get. It needs to be in the YYYY-MM-DD format |
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
Example
ba.data.getCardTransactions(cardID, "2020-01-01", "2020-02-01")
.then(transactionsResponse => {
if(transactionsResponse.status === "done") {
console.dir(transactionsResponse)
} else {
console.error("API Responded with an error")
console.dir(transactionsResponse)
}
})
.catch(error => {
console.dir(error)
})
Get Card Transactions Response
Credit Card Enhanced Transactions
Access the enhanced transactions of a given credit card.
Parameter | Type | Description |
---|---|---|
cardID | String | The id of a particular card Note: You can get this from calling getCards function |
fromDate | String | The start date of the transactions you want to get. It needs to be in the YYYY-MM-DD format |
toDate | String | The end date of the transactions you want to get. It needs to be in the YYYY-MM-DD format |
type | String | Specify transactions type.enriched -> Each transaction object will have category and brandDetails properties.categorized -> Each transaction object will have category property.default -> category and brandDetails won't be included in the response. |
clientHeaders | Object Optional | More details |
clientBody | Object Optional | More details |
ba.data.getCardEnhancedTransactions(cardID, "2020-01-01", "2020-02-01", "categorized")
.then(transactionsResponse => {
if(transactionsResponse.status === "done") {
console.dir(transactionsResponse)
} else {
console.error("API Responded with an error")
console.dir(transactionsResponse)
}
})
.catch(error => {
console.dir(error)
})
Note
Date range of the transactions that can be retrieved varies for each bank. The range supported by the users bank is shown in the response parameter
transactionRange
ofGet Accounts Metadata
endpoint. If the date range you provide is bigger than thetransactionRange
you'll getINVALID_DATE_RANGE
error.To make sure you always send a valid date range you should check the transactionsRange.
Example:function getTransactionsForNumberOfMonths(numberOfMonths) { let days = numberOfMonths * 30; let dayMillis = 24*60*60*1000; ba.metadata.getAccounts().then(payload => { let transactionsRange = payload.accountsMetadata.transactionRange; let unit = transactionsRange?.unit; let value = transactionsRange?.value; if(unit == null || value == null) { //Can't check transactions range.. } else { var multiplier = 1; if (unit == "years") { multiplier = 365; } else if (unit == "months") { multiplier = 30; } else { multiplier = 1; } var transactionRangeValueInDays = value * multiplier; var fromDate = new Date(); if(transactionRangeValueInDays > days) { //numberOfMonths is within the transaction range fromDate = new Date(Date.now() - (days * dayMillis)); } else { //numberOfMonths is NOT within the transaction range, so we query only for the allowed range fromDate = new Date(Date.now() - (transactionRangeValueInDays * dayMillis)); } let toDate = new Date(); ba.data.getAccounts().then(payload => { let account = payload.accounts[0]; ba.data.getTransactions(account.id, fromDate.toISOString().split('T')[0], toDate.toISOString().split('T')[0]).then(transactions => { }).catch(e => { }); }); } }); }
Updated over 1 year ago