Wire API (US only)
Wire API provides all the functionality required to manage beneficiaries and initiate payments on your user's behalf.
The Payment APIs are:
- CreateWireTransfer - The easiest and most efficient way to facilitate payments.
- [Not required when using CreateWireTransfer] Get Wire beneficiaries - Retrieve beneficiaries from the bank account.
- [Not required when using CreateWireTransfer] Create Wire Beneficiary - Create a beneficiary into the bank account.
- [Not required when using CreateWireTransfer] CreateWireTransferToExistingBeneficiary - Initiate a transfer to an existing wire beneficiary only.
createWireTransfer [Recommended]
createWireTransfer
automatically takes care of all requirements for adding a beneficiary as well as initiating a transfer. We recommend using createWireTransfer
to initiate a payment.
you can send money from
an account to
an account with a specific amount
.All 3 variables are optional.
Parameters
NoteBehavior of the SDK depends on the parameters that will be set.
Parameter | Description |
---|---|
toBeneficiary | Information of the recipient beneficiary. |
fromAccount | Account from where the amount must be transferred. If you don't set a from account, SDK will simply display a popup screen for your user to pick the account from our UI. |
amount | Amount to be transferred. If you don't set an amount SDK will display a screen with a numpad screen for your user to enter the amount in. |
remark | A message associated with the transfer. |
Example - WhenfromAccount
Is Not Specified
DapiLineAddress lineAddress = DapiLineAddress(
line1: "Line1",
line2: "Line2",
line3: "Line3"
);
DapiWireBeneficiary beneficiary = new DapiWireBeneficiary(
address: lineAddress,
accountNumber: "1234567654321",
name: "TestAccount",
country: "US",
receiverType: "retail",
routingNumber: "953347241",
nickname: "Nickname",
receiverAccountType: "checking",
firstName: "John",
lastName: "Doe",
zipCode: "72305",
state: "Arkansas",
city: "Conway");
await connection.createWireTransfer(null, beneficiary, 100, "remark");
The SDK shows UI for the user to select an account to send money from
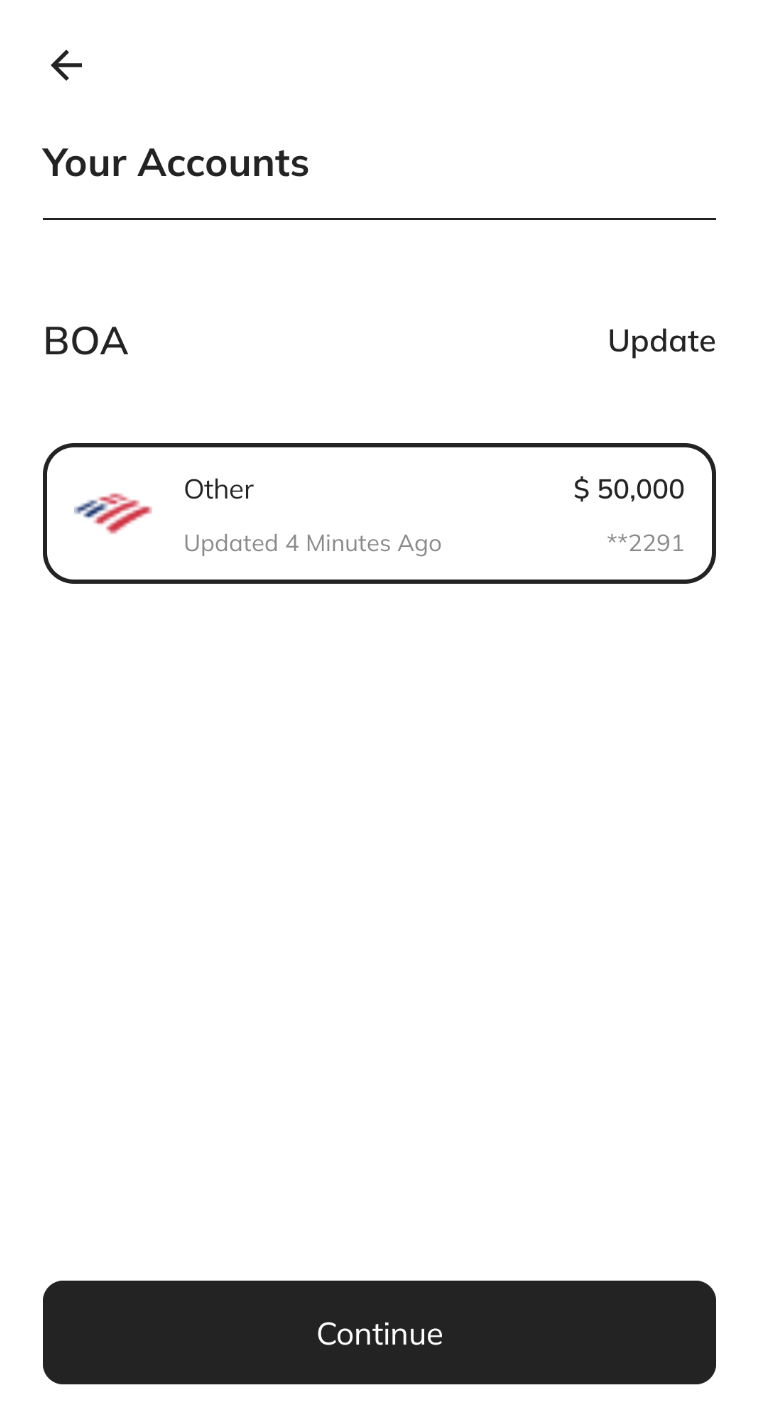
Account Selection Screen
Example - Whenamount
Is Not Specified
DapiLineAddress lineAddress = DapiLineAddress(
line1: "Line1",
line2: "Line2",
line3: "Line3"
);
DapiWireBeneficiary beneficiary = new DapiWireBeneficiary(
address: lineAddress,
accountNumber: "1234567654321",
name: "TestAccount",
country: "US",
receiverType: "retail",
routingNumber: "953347241",
nickname: "Nickname",
receiverAccountType: "checking",
firstName: "John",
lastName: "Doe",
zipCode: "72305",
state: "Arkansas",
city: "Conway");
await connection.createWireTransfer(account, beneficiary, 0, "remark");
The SDK shows UI for the user to enter the amount to send
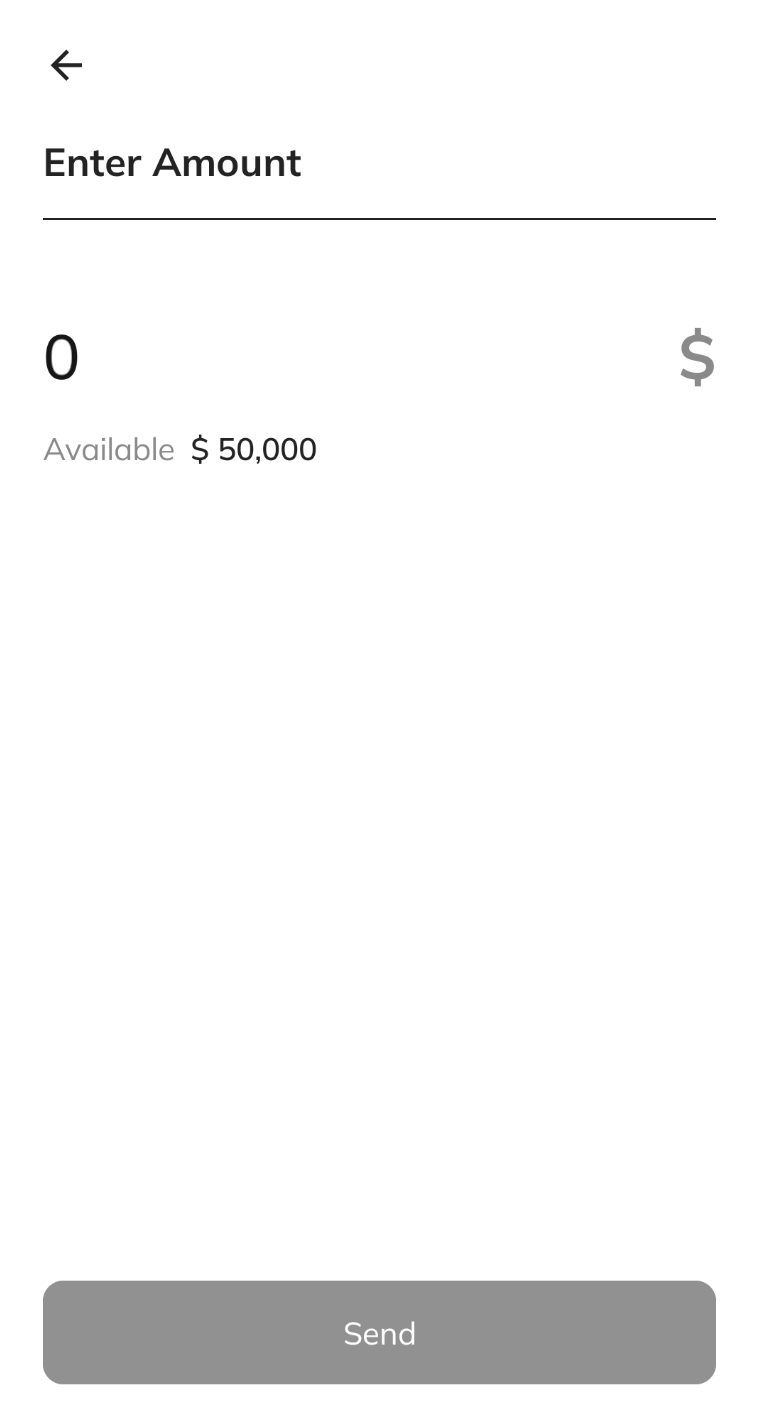
Amount Screen
Example - Whenamount
and fromAccount
Are Not Specified
DapiLineAddress lineAddress = DapiLineAddress(
line1: "Line1",
line2: "Line2",
line3: "Line3"
);
DapiWireBeneficiary beneficiary = new DapiWireBeneficiary(
address: lineAddress,
accountNumber: "1234567654321",
name: "TestAccount",
country: "US",
receiverType: "retail",
routingNumber: "953347241",
nickname: "Nickname",
receiverAccountType: "checking",
firstName: "John",
lastName: "Doe",
zipCode: "72305",
state: "Arkansas",
city: "Conway");
await connection.createWireTransfer(null, beneficiary, 0, "remark");
The SDK shows UI for the user to select an account to send money from then navigate to the amount screen to enter the amount to send
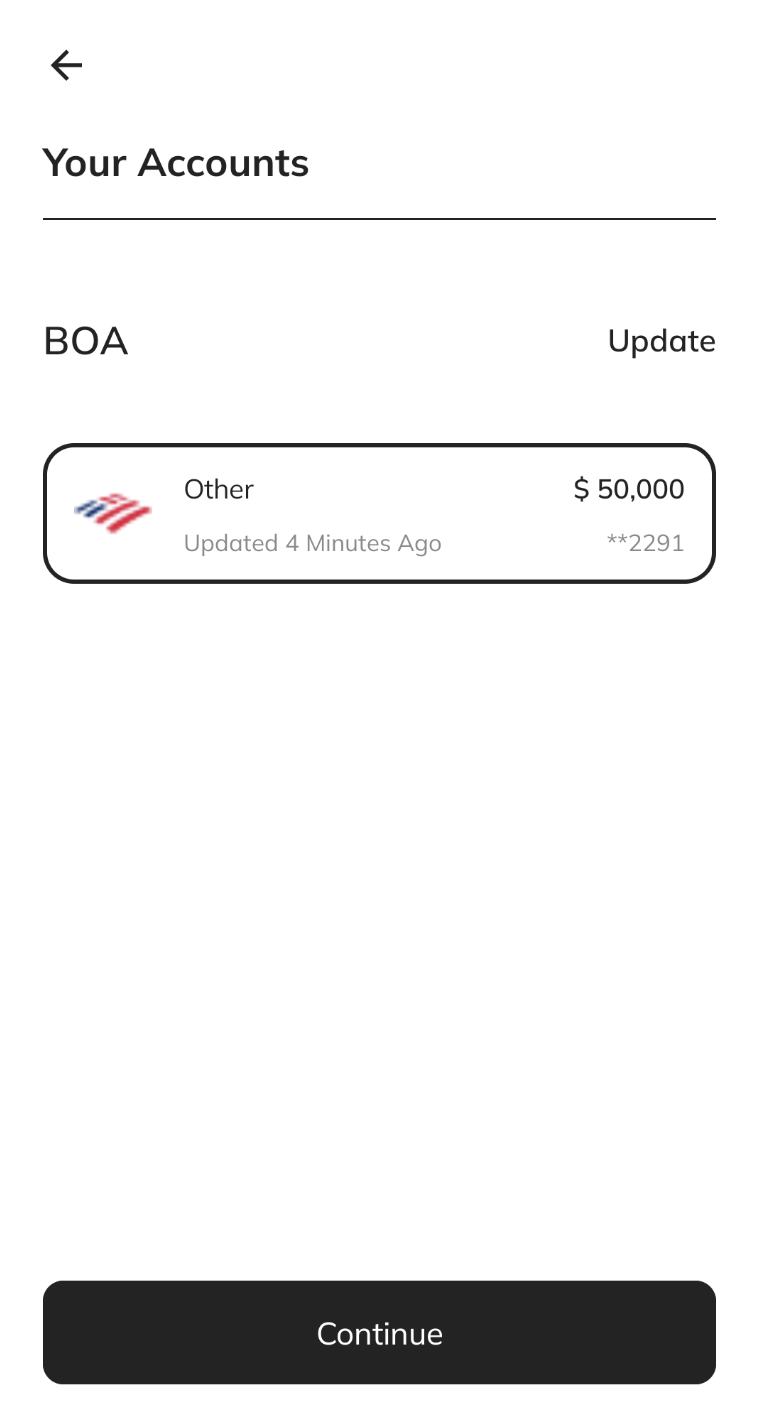
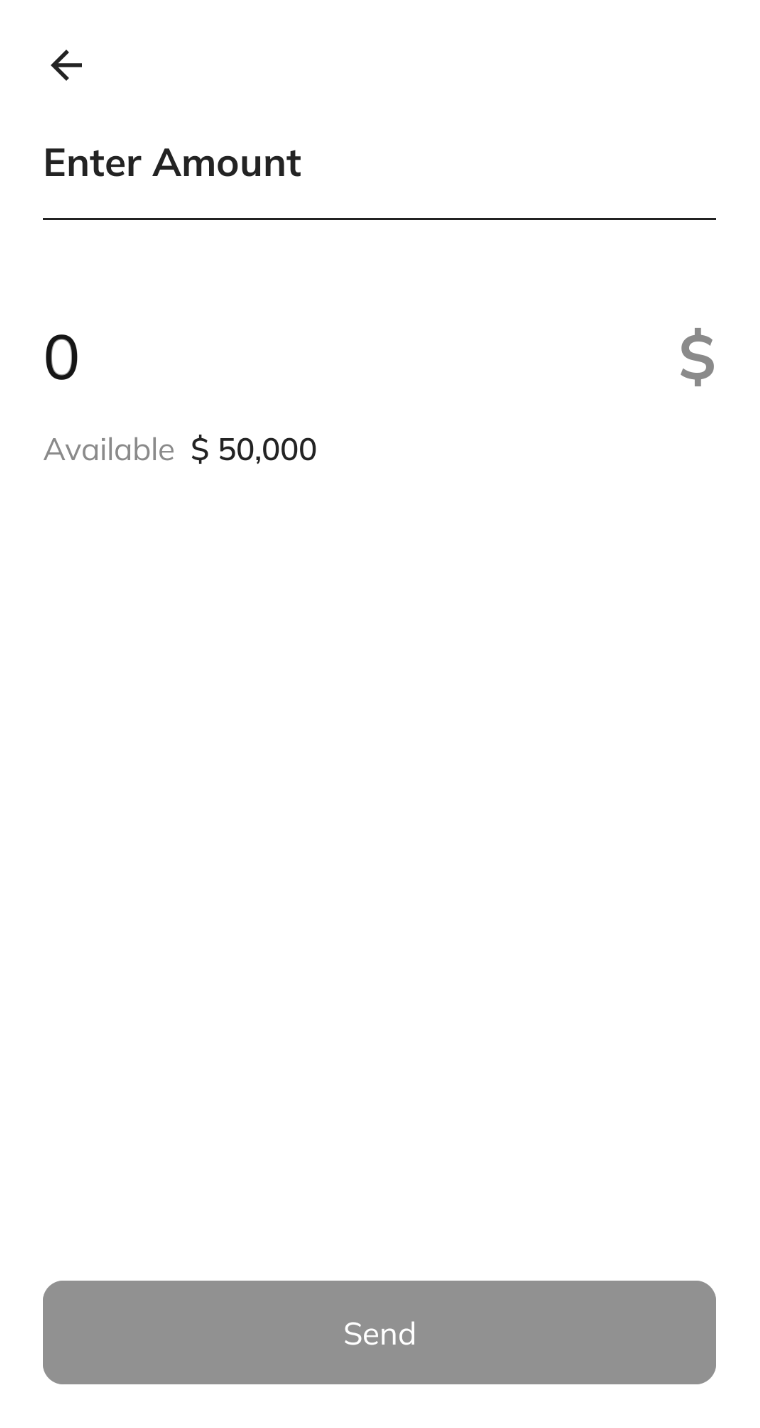
createWireTransferToExistingBeneficiary
NoteIf you use
createWireTransferToExistingBeneficiary
you have to implement bank processing logic and validations on your end. You will require the use of the following APIs to process a transaction:
- Get Account
- Create Wire Beneficiary
- Get Wire Beneficiaries
createWireTransfer
abstracts all these requirements. There is no need to implement bank processing logic or validations. Dapi recommends usingcreateWireTransfer
to initiate a payment.
A method for sending money to an existing wire beneficiary.
Parameters
Parameter | Description |
---|---|
fromAccount | Account from where the amount must be transferred. |
toBeneficiaryID | The id of the wire beneficiary to which the money must be transferred. Obtain by connection.getWireBeneficiaries() |
amount | Amount to be transferred |
Example
await connection.createWireTransferToExistingBeneficiary(
account,
beneficiaryID,
99.0,
"remark");
Beneficiary Not Activated error on SandboxThis error occurs If you make a transfer on Sandbox to wrong or random beneficiary details, instead you should make the beneficiary details to be of another sandbox user.
To get the beneficiary details of a sandbox user you can do the following:
1- Open your app on the dashboard and navigate to the Sandbox tab. You'll see the Sandbox Users table.
2- Click an Account from the Accounts column.
3- A dialog shows up containing beneficiary details.
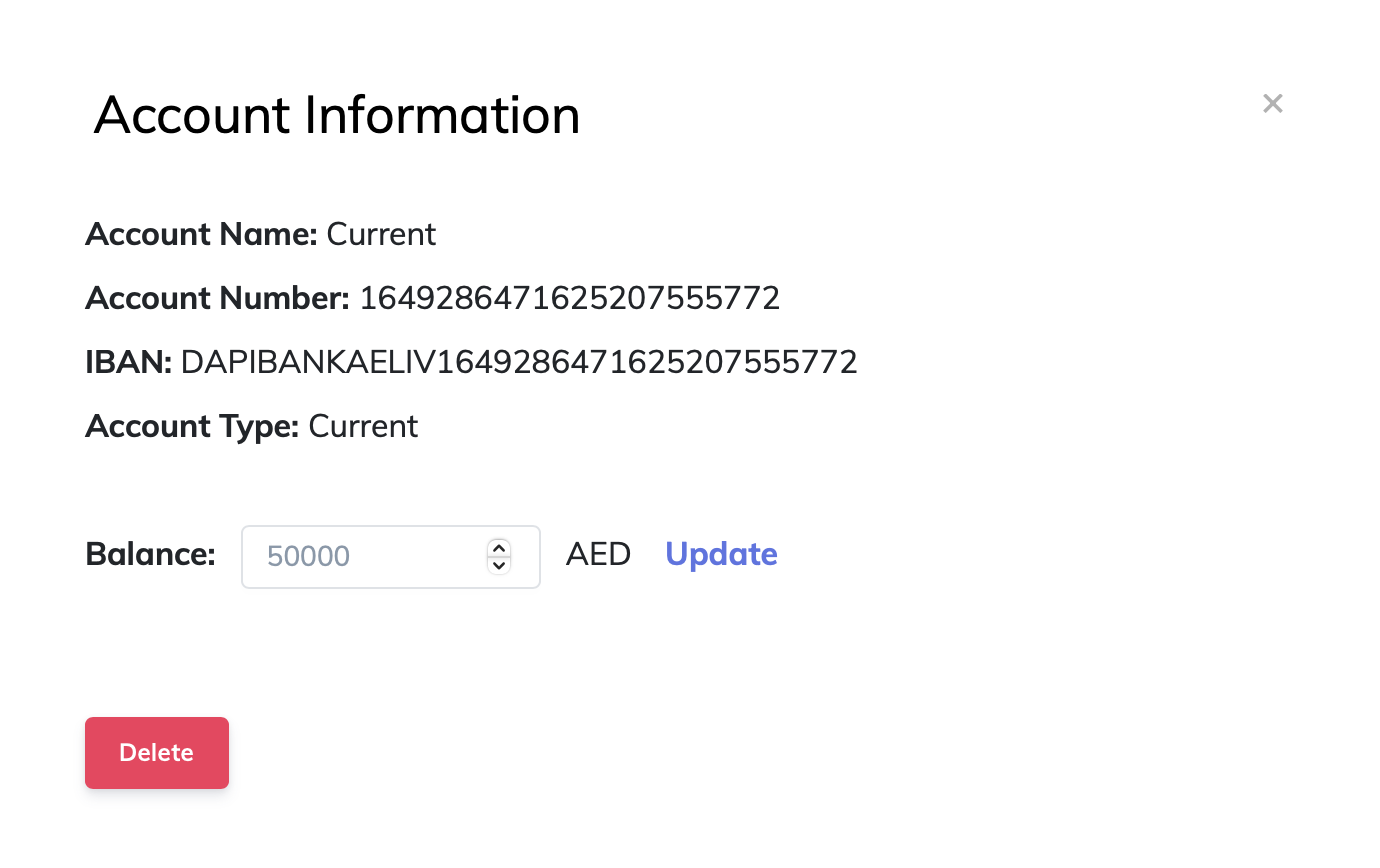
Sandbox Account Beneficiary details
createWireBeneficiary
A method for adding a new wire beneficiary
Parameters
Parameter | Description |
---|---|
beneficiary | Information of the wire beneficiary to add. |
Example
DapiLineAddress lineAddress = DapiLineAddress(
line1: "2400 bruce street UCA stadium park bld 6",
line2: "",
line3: ""
);
DapiWireBeneficiary beneficiary = new DapiWireBeneficiary(
address: lineAddress,
accountNumber: "1234567654321",
name: "TestAccount",
country: "US",
receiverType: "retail",
routingNumber: "953347241",
nickname: "Nickname",
receiverAccountType: "checking",
firstName: "John",
lastName: "Doe",
zipCode: "72305",
state: "Arkansas",
city: "Conway");
await connection.createWireBeneficiary(beneficiary);
getWireBeneficiaries
A method for obtaining registered wire beneficiaries
Parameters
Method does not receive any parameter.
Example
await connection.getWireBeneficiaries();
Updated 15 days ago