iOS SDK Configuration
This page covers how to configure the iOS SDK to be a part of your system.
Create SDK Configurations
DAPIConfigurations
is a singleton object that you can set once or update any time you want to add or modify the configurations. You can change the following configurations:
- Countries (countries that your users will have access to)
- Extra Fields (you can send custom body parameters or headers along the API calls)
- Result screens (whether result screens are displayed or not)
- Theme (Light or Dark Mode)
- Language (English and Arabic)
- Enable Network Logging
//Full example
let configurations = DAPIConfigurations(countries: [.us, .ae, .eg])
configurations.showTransferSuccessfulResult = true
configurations.showTransferErrorResult = false
configurations.endPointExtraBody = [.all: ["key": "Value"]]
configurations.endPointExtraHeaderFields = [.all: ["key": "value"]]
configurations.enableNetworkingLogging = true
Dapi.shared.configurations = configurations
// Update headers and body for a specific API endpoint
Dapi.shared.configurations.endPointExtraBody = [.identity: ["identityObject": [ "Key2":"Value" ] ]]
Dapi.shared.configurations.endPointExtraHeaderFields = [.identity: ["identityKey": "Value"]]
Dapi.shared.uiCustomization.theme = .dynamic
Dapi.shared.uiCustomization.primaryColor.lightModeHexaCode = "#000000"
Dapi.shared.configurations.language = .en
DAPIConfigurations *configurations = [[DAPIConfigurations alloc] init];
configurations.showTransferSuccessfulResult = YES;
configurations.showTransferErrorResult = YES;
configurations.enableNetworkingLogging = YES;
configurations.language = DAPILanguageEn;
[DPC.sharedInstance setCountries: [NSArray arrayWithObjects:@"AE",@"US", nil]] ;
[DPC.sharedInstance setEndpointExtraBody:@{DPCEndpointGetCards: @{@"key": @"value"}}];
[DPC.sharedInstance setEndpointExtraHeaderFields:@{DPCEndpointGetCards: @{@"key": @"value"}}];
[DPC.sharedInstance setConfigurations:configurations];
DAPIUICustomization *uiCustomization = [[DAPIUICustomization alloc] init];
[uiCustomization setTheme: DAPIThemeDynamic];
// [uiCustomization.primaryColor setLightModeHexaCode:@"#000000"];
// [uiCustomization.primaryColor setDarkModeHexaCode:@"FFFFFF"];
[DPC.sharedInstance setUICustomization:uiCustomization];
Set Supported Countries
Initialize DAPIConfigurations
with the countries
that you want to access on Dapi.
let configurations = DAPIConfigurations( countries : [.ae,.us] )
Dapi.shared.configurations = configurations
[DPC.sharedInstance setCountries: [NSArray arrayWithObjects:@"AE",@"US", nil]] ;
If you couldn't find the country that you support, use the Alpha-2
country codes from country codes
let configurations = DAPIConfigurations( countries : [.countryCode(code: "COUNTRY CODE"),.us] )
Dapi.shared.configurations = configurations
[DPC.sharedInstance setCountries: [NSArray arrayWithObjects:@"AE",@"US", nil]] ;
Add Extra Header Fields
You can add header fields to a specific API or all APIs using endPointExtraHeaderFields
Add Headers To All Endpoints
Dapi.shared.configurations.endPointExtraHeaderFields = [.all: ["HeaderKey1": "Value1",
"HeaderKey2": "Value2"]]
[DPC.sharedInstance setEndpointExtraHeaderFields:@{DPCEndpointAll: @{@"key": @"value"}}];
Add Headers To a Specific Endpoint
Dapi.shared.configurations.endPointExtraHeaderFields = [.identity: ["HeaderKey1": "Value1",
"HeaderKey2": "Value2"]]
[DPC.sharedInstance setEndpointExtraHeaderFields:@{DPCEndpointGetCards: @{@"key": @"value"}}];
Using
.all
and endpoint-specific headersScenario: If you use
endPointExtraHeaderFields
to set header fields to.all
endpoints and at the same time you set header fields to a specific endpointResult: The SDK will use the values set for the specific endpoint and ignore the values in
.all
.
Add Extra Body
You can add extra body to send to a specific endpoint or all endpoints using endPointExtraBody
The Extra body will look like UserExtraBody:{Key1:"Value1", "Key2": "Value2"}
in any request body.
Add Body To All Endpoints
Dapi.shared.configurations.endPointExtraBody =[.all: ["Key1": "Value1",
"Key2": "Value2"]]
[DPC.sharedInstance setEndpointExtraBody:@{DPCEndpointAll: @{@"key": @"value"}}];
Add Body To A Specific Endpoint
Dapi.shared.configurations.endPointExtraBody =[.identity: ["Key1": "Value1",
"Key2": "Value2"]]
[DPC.sharedInstance setEndpointExtraBody:@{DPCEndpointGetCards: @{@"key": @"value"}}];
Using
.all
and endpoint-specific body parametersScneario: If you use
endPointExtraBody
to set the extra body to.all
endpoints and at the same time you set extra body to a specific endpoint.Result: The SDK will use the values set for this specific endpoint and ignore the values in
.all
.
Show Transfer Result Screen
You can show the transfer result screen, by using showTransferErrorResult
to display the error and showTransferSuccessfulResult
to display the success screen.
The default value is Ture
for showTransferErrorResult
and showTransferSuccessfulResult
Dapi.shared.configurations.showTransferErrorResult = false
Dapi.shared.configurations.showTransferSuccessfulResult = false
DAPIConfigurations *configurations = [[DAPIConfigurations alloc] init]; // [DPC.sharedInstance configurations];
configurations.showTransferSuccessfulResult = YES;
configurations.showTransferErrorResult = YES;
[DPC.sharedInstance setConfigurations:configurations];
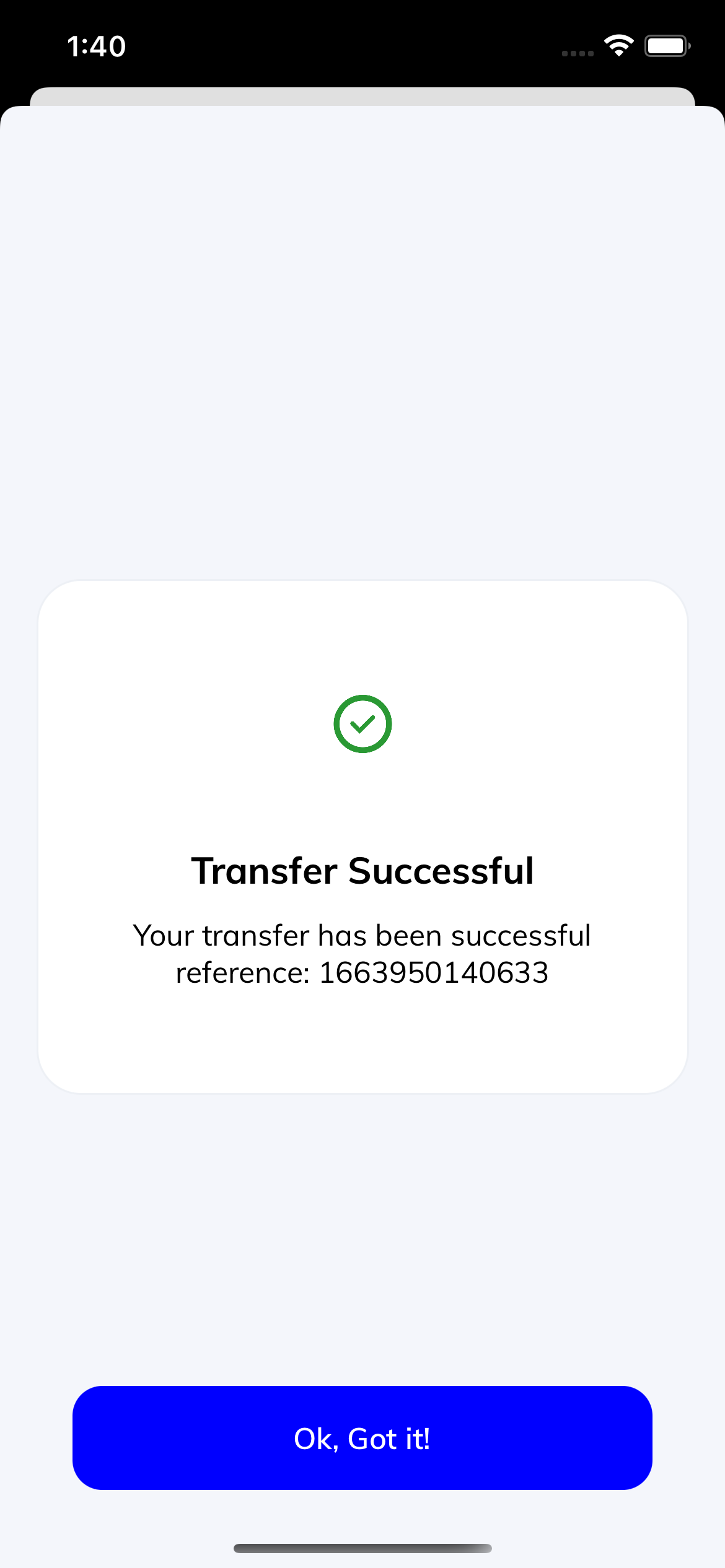
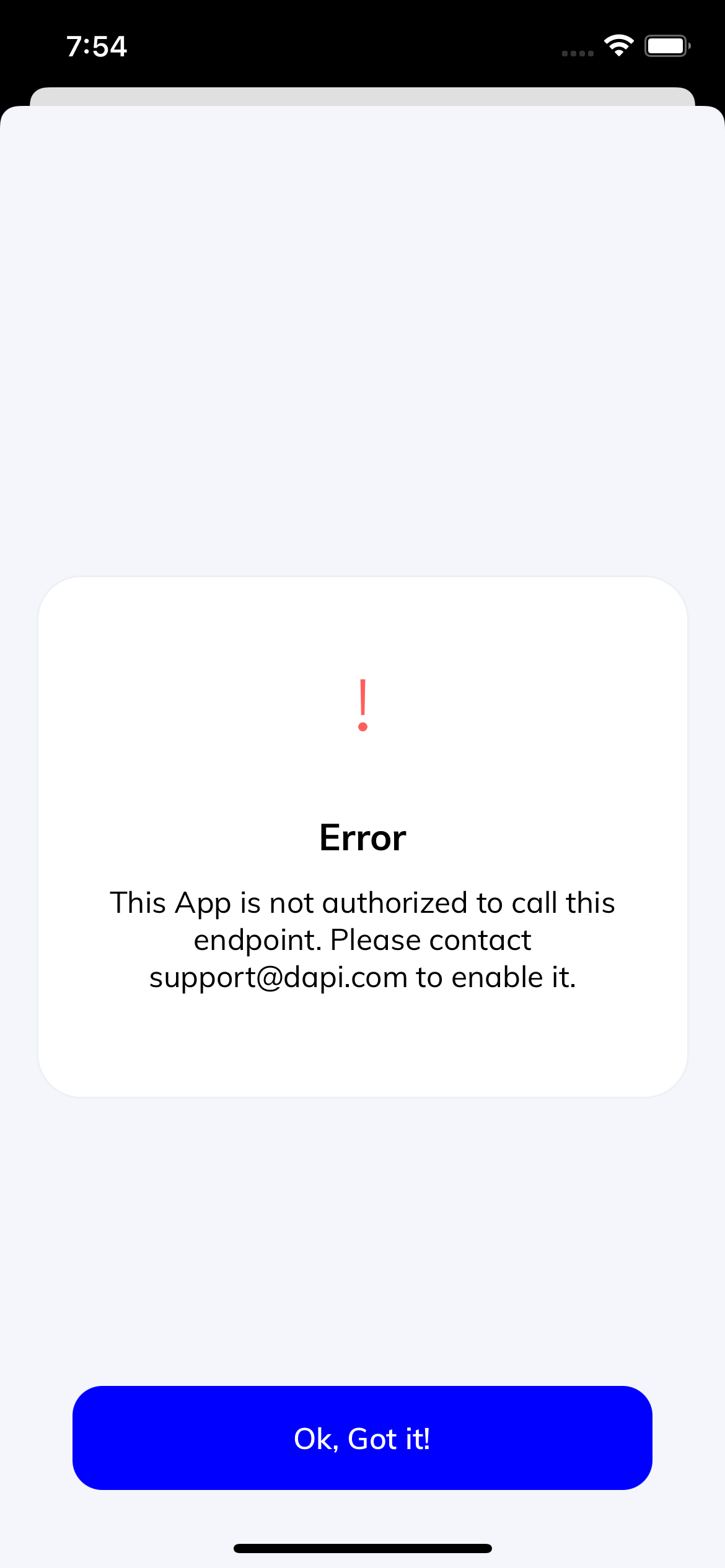
Customize UI Color & Theme
You can customize how DAPI appears in your app to match your design and brand palette.
To Customize UI Color & Theme, you should use SDK version 2.0.0 and above.
Set SDK Themes
The SDK UI support two color themes: light and dark.
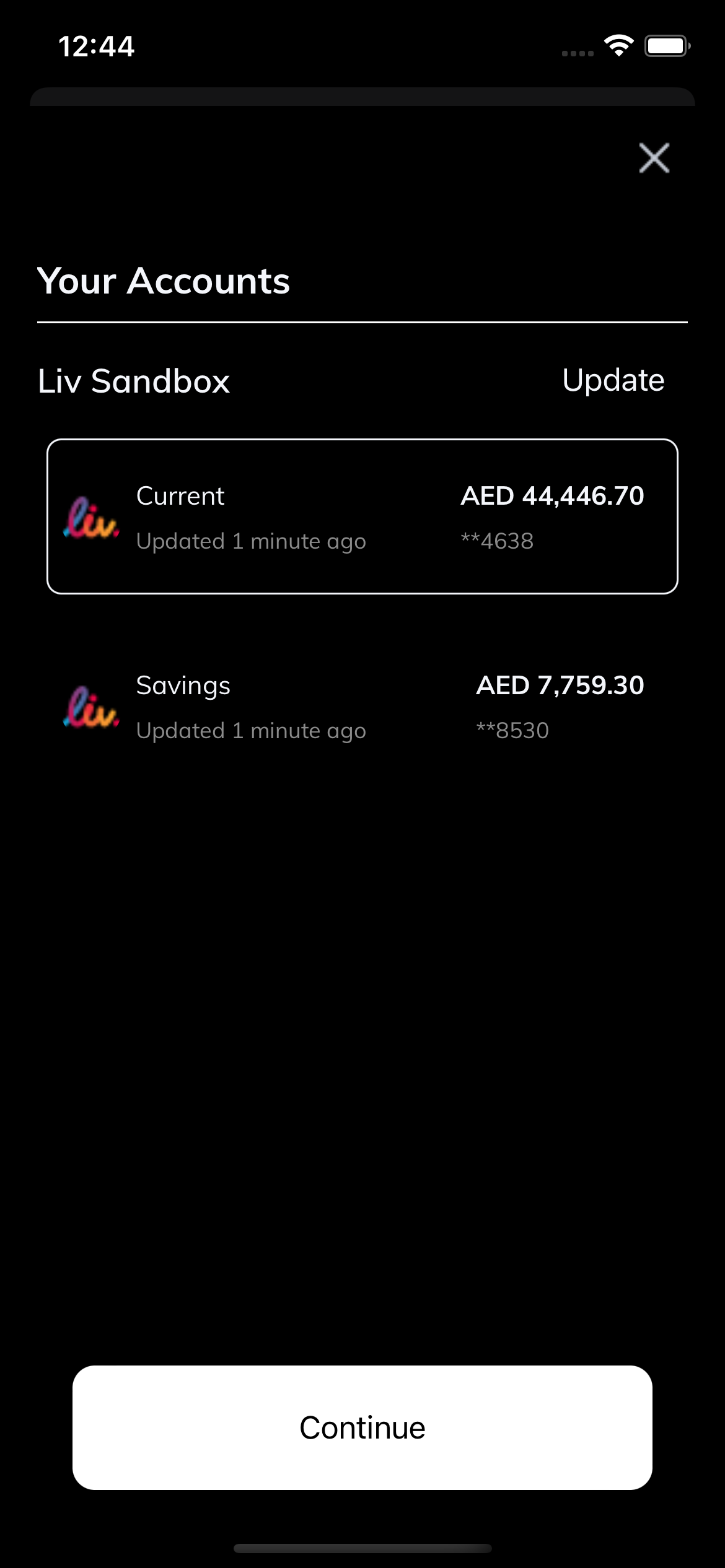
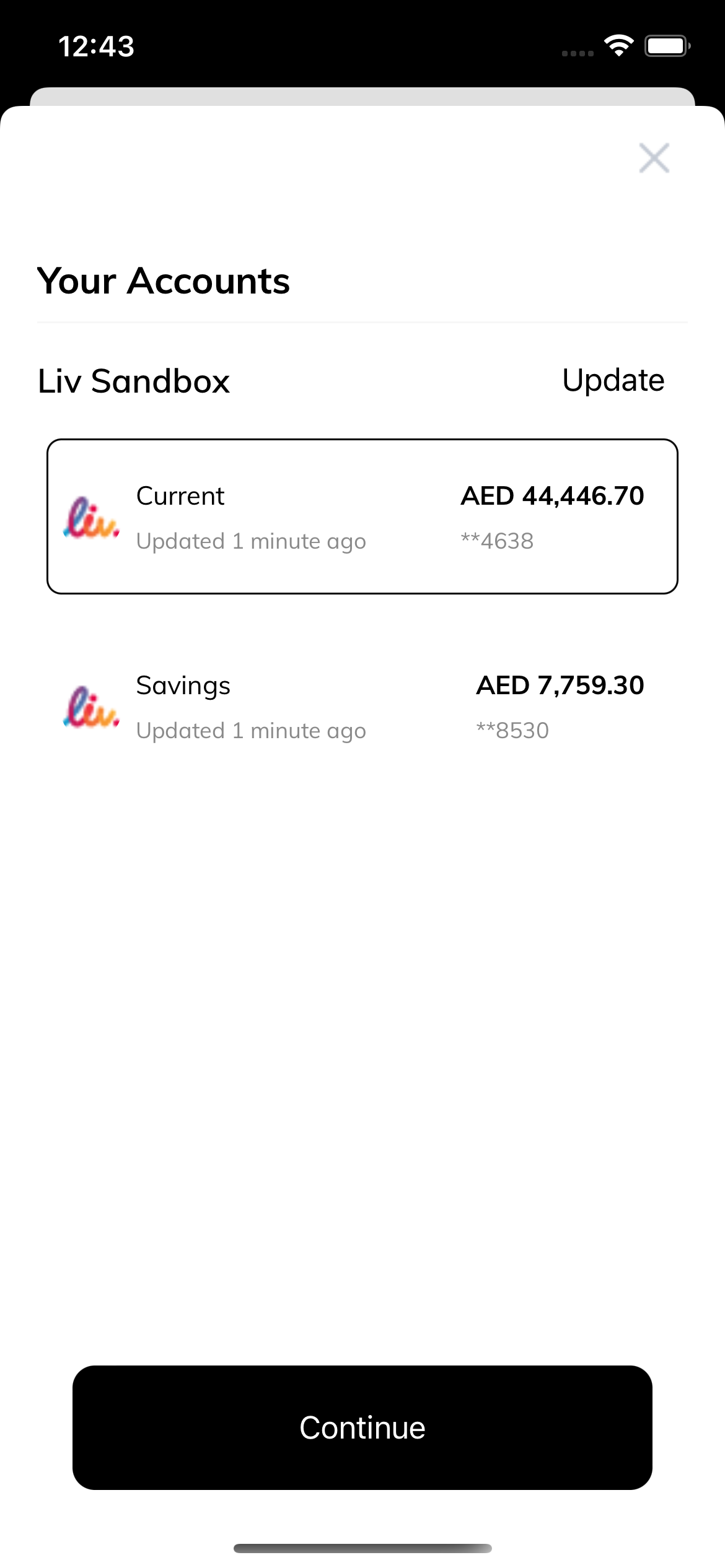
You can set a theme with dark, light, or dynamic.
Dynamic means that SDK follows the device theme.
Dapi.shared.uiCustomization.theme = .dark
Dapi.shared.uiCustomization.theme = .light
Dapi.shared.uiCustomization.theme = .dynamic
DAPIUICustomization *uiCustomization = [[DAPIUICustomization alloc] init]; //or [DPC.sharedInstance uiCustomization];
[uiCustomization setTheme: DAPIThemeDark];
[uiCustomization setTheme: DAPIThemeLight];
[uiCustomization setTheme: DAPIThemeDynamic];
[DPC.sharedInstance setUICustomization:uiCustomization];
Set The Primary Color
You can also set the color of UI elements that indicate interactivity, like links, or a call to action, like buttons. You can set the primaryColor
separately for light and dark modes.
Dapi.shared.uiCustomization.primaryColor.lightModeHexaCode = "#000000"
Dapi.shared.uiCustomization.primaryColor.darkModeHexaCode = "FFFFFF"
DAPIUICustomization *uiCustomization = [[DAPIUICustomization alloc] init]; // Or [DPC.sharedInstance uiCustomization];
[uiCustomization.primaryColor setLightModeHexaCode:@"#000000"];
[uiCustomization.primaryColor setDarkModeHexaCode:@"FFFFFF"];
[DPC.sharedInstance setUICustomization:uiCustomization];
Set The Language
The SDK supports two languages: Arabic and English.
Use the following code to set the SDK language
Dapi.shared.configurations.language = .en
//or
Dapi.shared.configurations.language = .ar
DAPIConfigurations *configurations = [[DAPIConfigurations alloc] init]; // [DPC.sharedInstance configurations];
configurations.language = DAPILanguageEn;
configurations.language = DAPILanguageAr;
[DPC.sharedInstance setConfigurations:configurations];
Enable Network Logging
Enable network logging to log in the console the full request information on the requests that fire from the SDK.
Using Scheme Arguments [Recomeded]
- Open
Product
->Scheme
->Edit Scheme
->Arguments
- Add
DAPI_NETWORK_LOGGIN_ENABLED
environment variable with valueYES
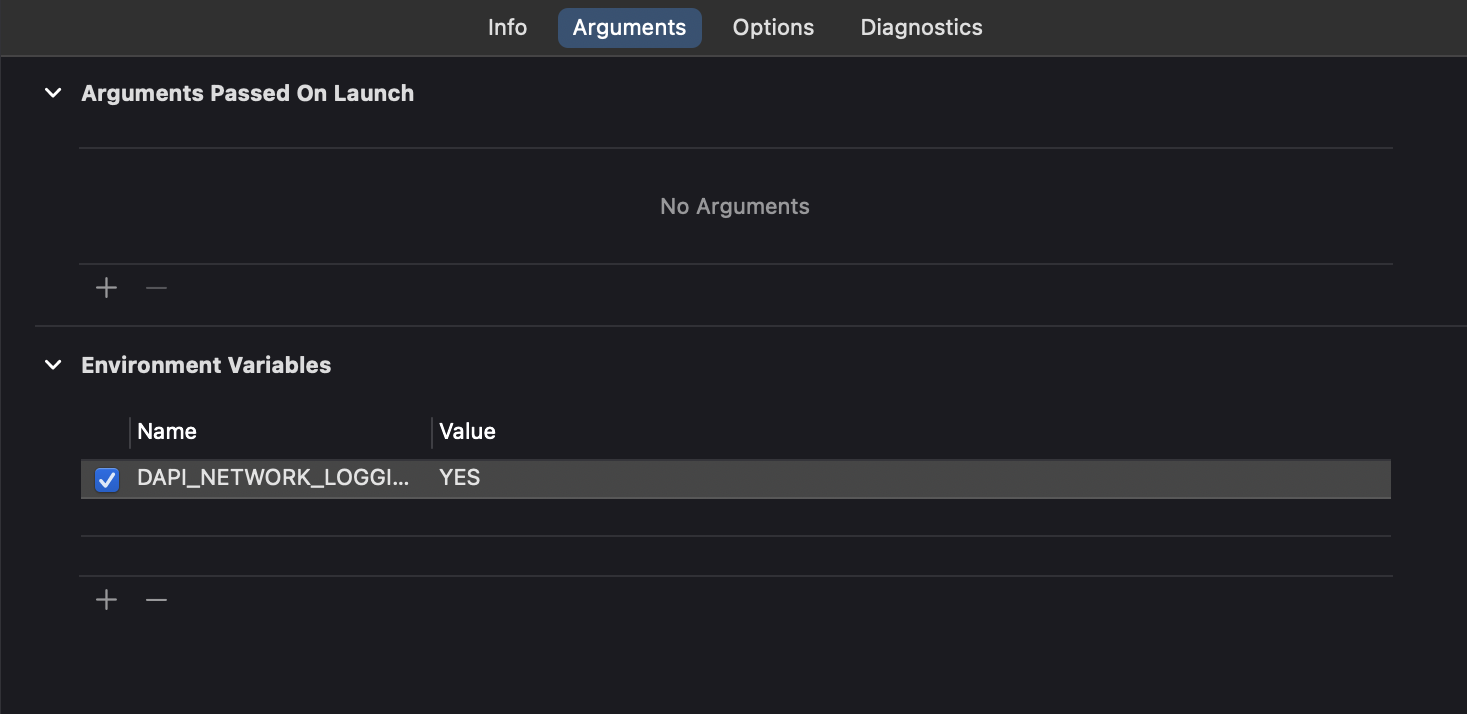
Using SDK Configurations
Dapi.shared.configurations.enableNetworkingLogging = true
DAPIConfigurations *configurations = [[DAPIConfigurations alloc] init]; // [DPC.sharedInstance configurations];
configurations.enableNetworkingLogging = YES;
[DPC.sharedInstance setConfigurations:configurations];
Updated over 1 year ago