Create Bank Connection
Create Bank Connection
Let's now create a connection object. A connection represents a user's connection to a bank. So if they authenticate and login, through Dapi, to 2 banks there will be 2 connections.
Since you don't know yet which bank the user will choose, you will just display the connect page. The user has to then pick the bank and enter their credentials.
Dapi.presentConnect();
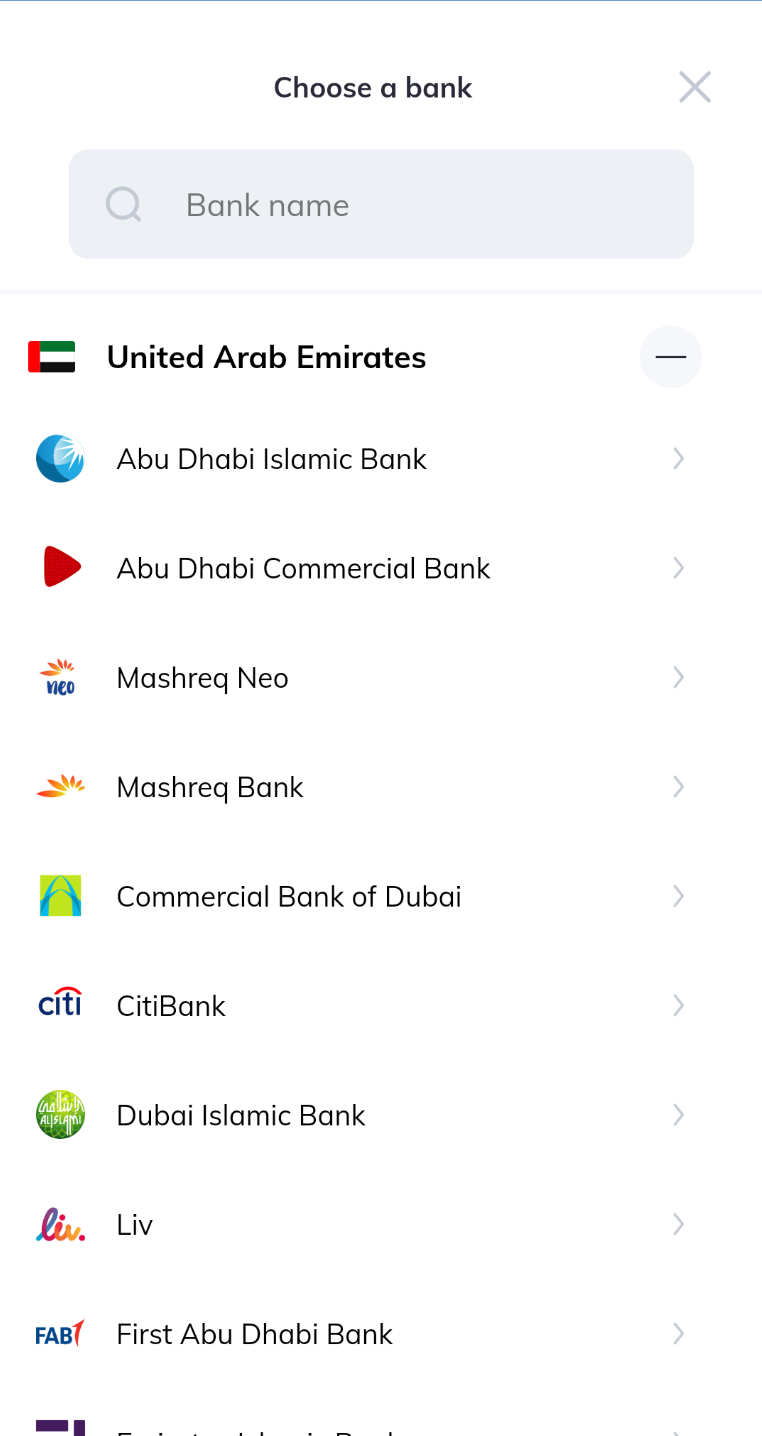
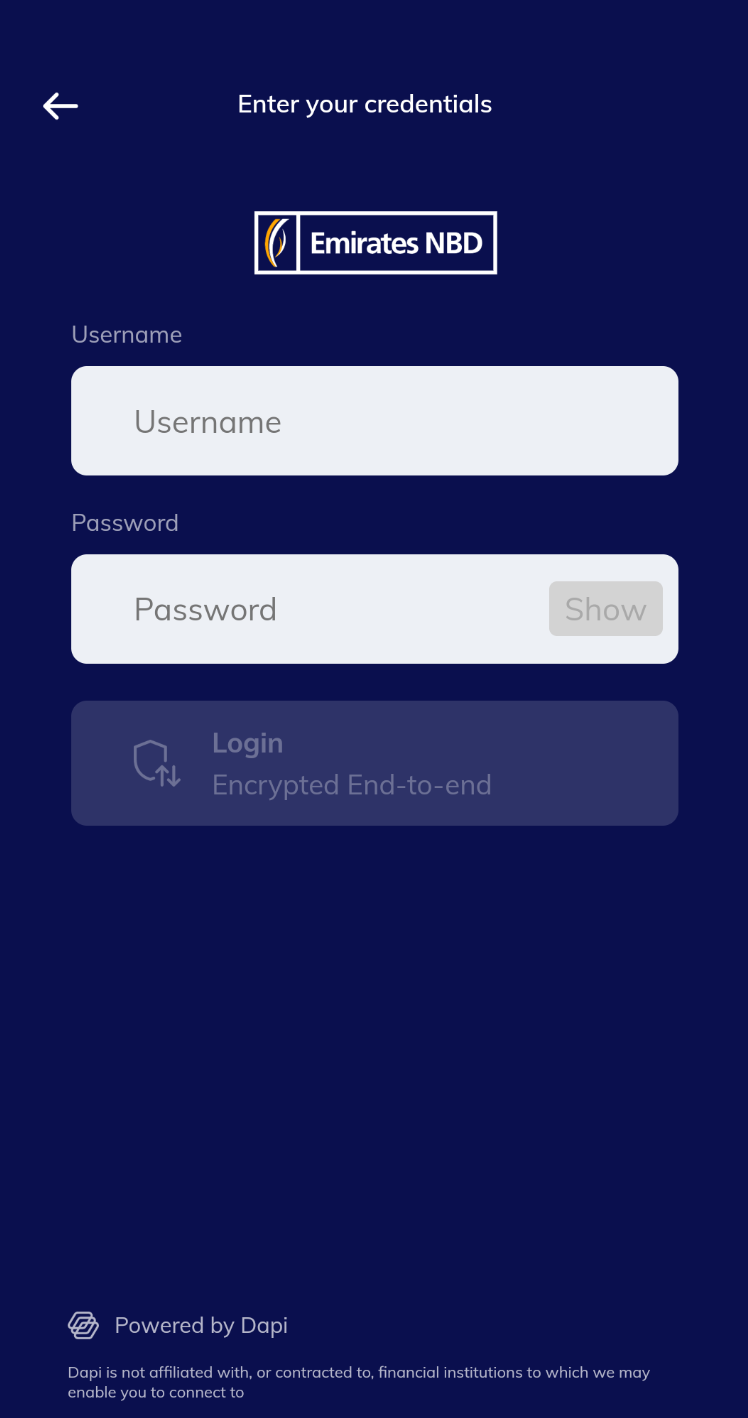
Add listeners to handle a successful or a failed connection attempt.
Dapi.onConnectionSuccessful.listen((m) => print("CONNECTION_SUCCESSFUl"));
Dapi.onConnectionFailure.listen((m) => print("CONNECTION_FAILED"));
That's it. You can now try to run your app on the emulator and call the presentConnect
function and see Dapi in action!
Create Sandbox User
You can create users for sandbox environment to test your integration with Dapi instead of connecting your real accounts
Navigate to Dashboard -> Apps -> Your App -> Sandbox -> Create a Sandbox User
Enter the following details and click Submit.
- Sandbox Bank: select a bank from the dropdown list
- Sandbox Username : username of your choice
- Sandbox Password : password of your choice
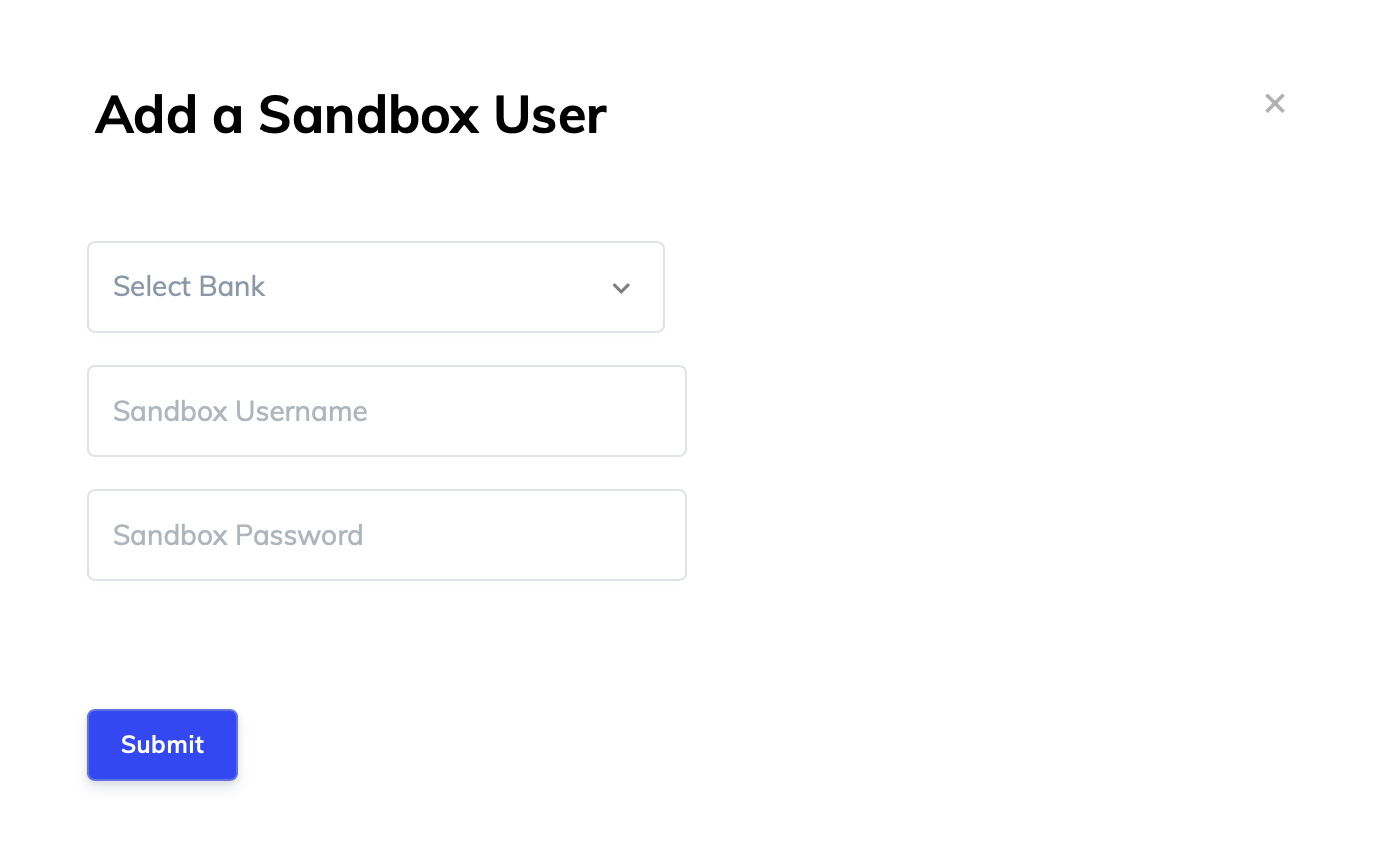
In order to add accounts to a sandbox user, click the NEW button on the Accounts column and provide the following information:
- Acount Name: user's account name (You can see it on the Account Information dialog when you click an account from the Sandbox Users table.)
- Account Number: user's account number (You can see it on the Account Information dialog)
- IBAN: user's IBAN (You can see it on the Account Information dialog)
- Account Type: choose a value from the dropdown
- Balance: set a starting balance for the account
- Currency: choose the currency for the account from the dropdown
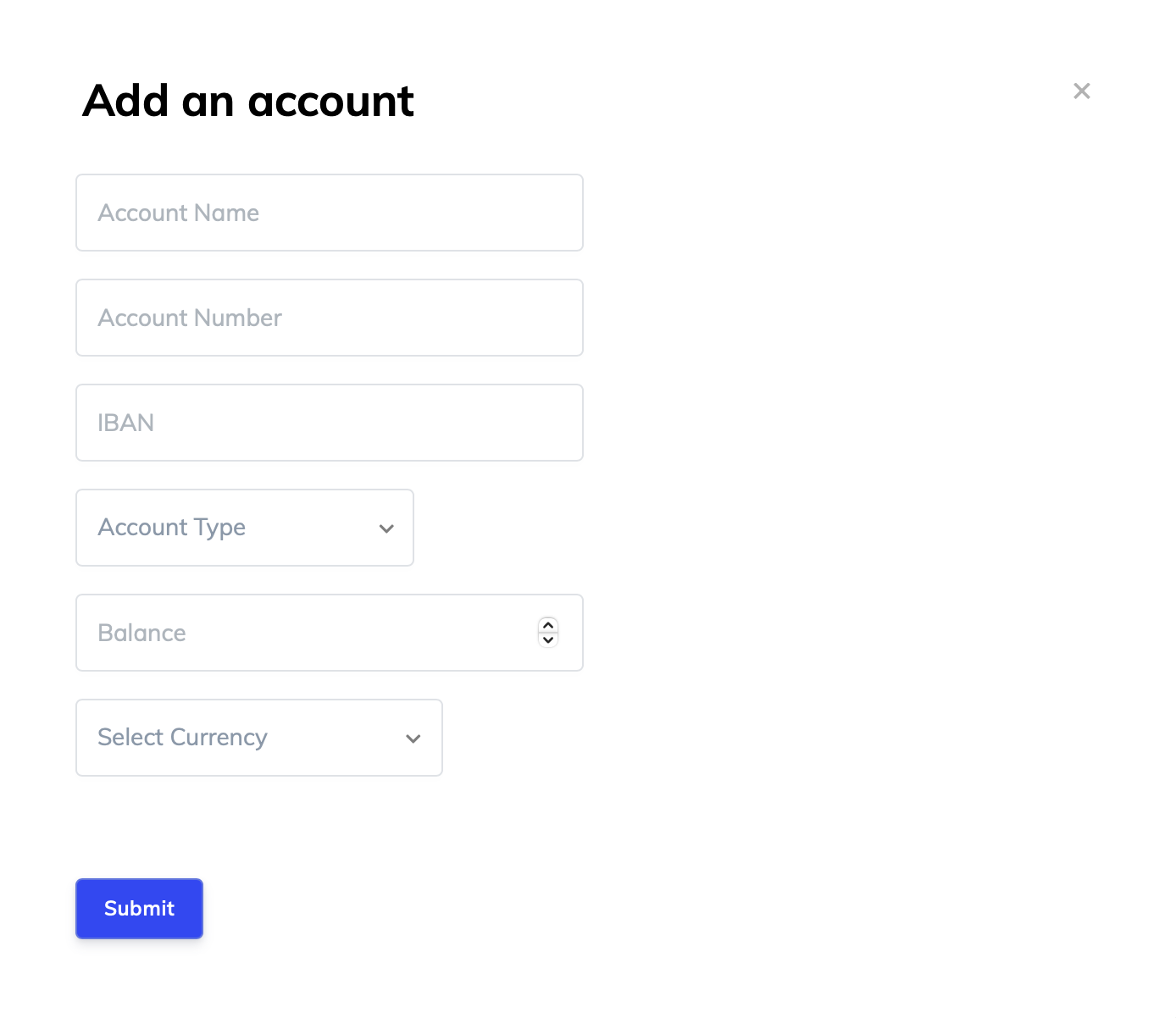
Get Cached Connections
When you authenticate and login through Dapi, a DapiConnection object is created and stored in the local cache containing information about your connection to the bank, so that you don't have to authenticate and login again every time you open the app.
Dapi.getConnections() returns the list of connections in cache.
await Dapi.getConnections()
Dismiss Connect
Closes the presented Connect UI
Parameters
Method does not receive any parameter.
Example
Dapi.dismissConnect();
getAccountsMetaData
A method for obtaining bank account metadata
Parameters
Method does not receive any parameter.
Example
await connection.getAccountsMetadata();
Get Parameters
A method for obtaining the connection parameters
Parameters
Method does not receive any parameter.
Example
await connection.getParameters();
Response
{
"bankId":"DAPIBANK_AE_ADCB",
"clientUserID":"clientID",
"color":{
"primaryColor":"#BD0000",
"secondaryColor":"#2A2B38"
},
"fullLogoPng":"https:\/\/cdn.dapi.com\/banks-full-logo\/ADCB.png",
"fullName":"Abu Dhabi Commercial Bank Sandbox",
"halfLogoPng":"https:\/\/cdn.dapi.com\/banks-horizontal-logo\/ADCB.png",
"miniLogoPng":"https:\/\/cdn.dapi.com\/banks-mini-logo\/ADCB.png",
"name":"ADCB Sandbox",
"tokenID":"23302e94-e463-450a-8794-52c6a19cd271",
"userID":"7x4orrsRTbIDXLcW2rgHbv1wVSsOOxHdRPg3v0SuSjH57LfPp5\/rFayQDnvnWnVg8C8XqaGaenWhN1gjMg6dzw==",
"userSecret":"rAaanOaptNFQ2ealZAZpcH3C8YgBIbiczDmOLZR0Y2M3a3wq4AlDtbRlqtEQtmLNarMh7\/J1xVr3xwaaTNATPU4r8IE4zSY6Xm+GOML8v+ArTAYIsHEEIaqdsLgblUTUhKlGYOUuDdWHAatc6aMGsdhC+AcpeS\/JkSc8KaqX6BgusOuD0rPVL46mdKiRvO7Vu7t8J0KU4KDc8nYQt2IYGiz778S1aYjQoHVCCSLeyoQuzPAdkaOpSweNiCihSzFNI5\/OR8YyQezcpHTdgj5gCD1cXg7\/zl9XWBD\/KCRV8ldDHt3rzMpJjXZUieDQ7nS92hWxHjruoVL10U6o3nyc6NubfePFjXy4voHda\/G227SE2yQ4NLBzWy3KdHNRtGjMNBHX9A93x0IrCJamOS5y+A3lTL1xONnqhw9QsWd+\/t8bN\/3hX2R18NiLT\/wKy017CRC7nFoCwwsmitTXYRf9ZyZVe9UexDlX4vGnWdsJc3xzHw87IJH4\/s+32YVWX+73yVfUYyfcETFuZ6tGDY7YZkgBxAnEDFP97o1F0E6SPS\/zq7GS3t+7bXVuyA291F1pvqccFjCoLGkXdYhXnrDpdGtw1+tYecKAa5CpFS+q54KuSHlHi4BFzVN9C5jtKt4xEAv7Z1a1mSzOLXlzPCdX+rmWF9mJDKrN0\/Ydb5pQPgg="
}
Create Connection
Add a new connection based on connection parameters.
Parameters
Parameter | Description |
---|---|
jsonConnectionParameters | DapiConnection's parameters, can be obtained using connection.getParameters() |
Example
await DapiConnection.create(jsonConnectionParams);
Updated about 1 month ago