Create Bank Connection
How to create a bank connection that is used for future API calls
Sandbox Environment Access
Our Sandbox environment allows you to simulate users and financial institutions with the full Dapi API workflow.
For more details check Get Started with Sandbox.
Create Bank Connection
Dapi Bank Connection
is a tokenized access to one bank account. The Bank Connection parameters have to be used during all API calls.
A Bank Connection
represents a user's connection to one bank. If the same user connects 2 banks on your application, there will be also 2 separate Bank Connection objects.
Bank Connection Using Connect Layer
All Bank Connections need to be established through Dapi's Connect Layer. Connect Layer is already embedded in the SDK and allows the users to choose the desired bank and provide their credentials.
Use DAPIConnectVC
to display the connection flow:
let connectVC = DAPIConnectVC()
connectVC.delegate = self
present(connectVC, animated: true, completion: nil)
DAPIConnectVC *connect = [[DAPIConnectVC alloc] init];
connect.delegate = self;
[self presentViewController:connect animated:true completion:nil];
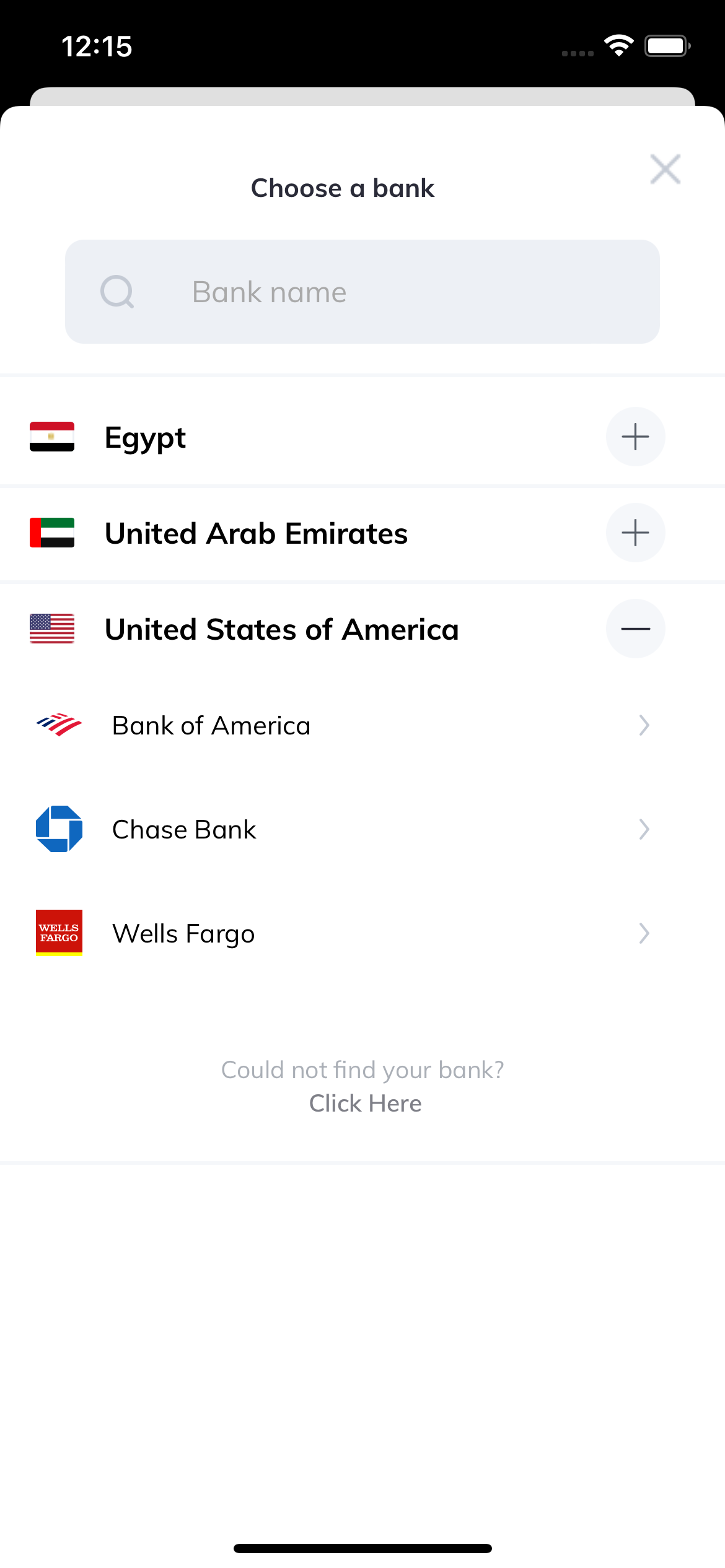
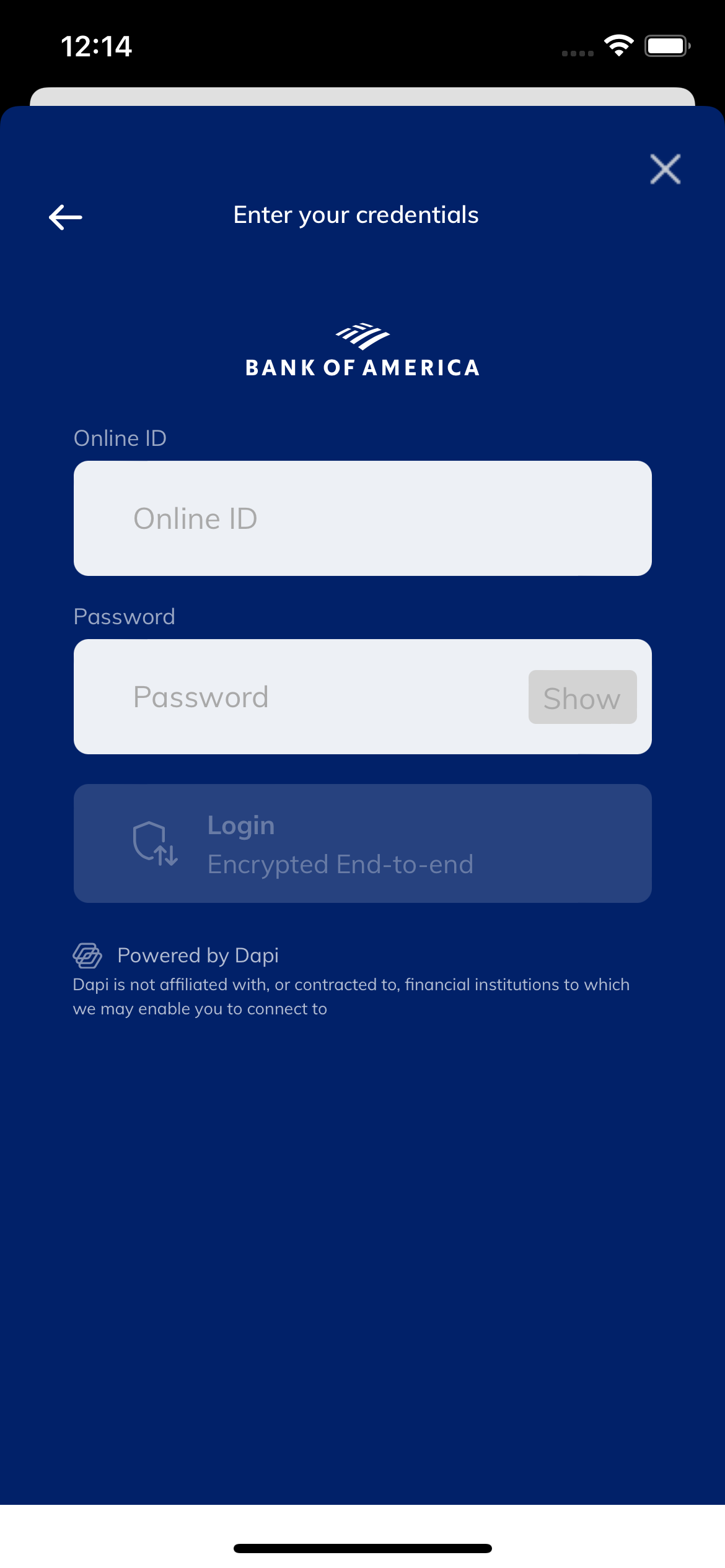
Use DAPIConnectDelegate
to handle a successful or a failed adding bank connection attempt.
extension ViewController: DAPIConnectDelegate {
///Tells the delegate that user successfully authenticated
func dapiConnect(_ dapiConnect: DAPIConnectVC,
didSuccessfullyConnectTo bankID: String?,
bankConnection: DAPIBankConnection) {
print(connection)
}
///Tells the delegate that bank connection failed with error message
func dapiConnect(_ dapiConnect: DAPIConnectVC,
didFailConnectingWith error: String) {
print(error)
}
///Tells the delegate that user closed `dapiConnect` ViewController
func dapiConnectUserDidCancel(_ dapiConnect: DAPIConnectVC) {
print("User Did Cancel ")
}
}
///Tells the delegate that bank connection failed with error message
- (void)dapiConnect:(DAPIConnectVC * _Nonnull)dapiConnect didFailConnectingWith:(NSString * _Nonnull)error {
NSLog(@"Connection Failed: %@", error);
}
///Tells the delegate that user successfully authenticated
- (void)dapiConnect:(DAPIConnectVC * _Nonnull)dapiConnect didSuccessfullyConnectTo:(NSString * _Nullable)bankID
bankConnection:(DAPIBankConnection * _Nonnull)bankConnection {
NSLog(@"Successful Connection: %@", bankConnection.userID);
}
///Tells the delegate that user closed `dapiConnect` ViewController
- (void)dapiConnectUserDidCancel:(DAPIConnectVC * _Nonnull)dapiConnect {
NSLog(@"ConnectUserDidCancel");
}
Bank Connection Using Connection Parameters
This approach can only be used if you are storing the connection parameters on your database.
Make sure that you have all of the required parameters in order to create a successful bank connection.
var parameters = [
"userID": "U6Kx3/if3Ko7FDg9ypVoZmzzEWFMzrsVjVA/PNYoyiPF8HGl0CvaSpgnsnVep49H8D2pDEROAc3L24P+s6ZfrQ==",
"tokenID": "f6335219-7466-44ef-8031-22e9739315cd",
"connectionID": "a6479d32-d062-41f6-abea-0f374ed7d677",
"clientUserID": "JohnDoe",
"userSecret": "userSecret",
"bankId": "DAPIBANK_AE_ENBD",
"name": "ENBD" ] as [String: Any]
Dapi.shared.createBankConnection(parameters: parameters) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiMetaData)
print(error.dapiErrorMessage)
}
}
NSDictionary *parameters = @{
@"userID": @"U6Kx3/if3Ko7FDg9ypVoZmzzEWFMzrsVjVA/PNYoyiPF8HGl0CvaSpgnsnVep49H8D2pDEROAc3L24
@"tokenID": @"f6335219-7466-44ef-8031-22e9739315cd",
@"connectionID": @"a6479d32-d062-41f6-abea-0f374ed7d677",
@"clientUserID": @"JohnDoe",
@"userSecret": @"userSecret",
@"bankId": @"DAPIBANK_AE_ENBD",
@"name": @"ENBD"
};
[Dapi.shared createBankConnectionWithParameters:parameters
completion:^(DAPIBankConnection * _Nullable connection,
NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@", connection);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Get Cached Connections
When the user authenticates and logs in through Dapi, a DAPIBankConnection object is automatically created and stored in the local cache. DAPIBankConnection contains information about one bank connection.
When using a cached DAPIBankConnection, the user does not need to authenticate through the Connect Layer flow again.
Every DAPIBankConnection is associated with app_key
, run_environment
and client_ID
let connections = Dapi.shared.bankConnections
let connection = connections.first(where: {$0.bankInfo.bankFullName == "Royal Bank of Canada" })
NSArray<DAPIBankConnection *> *connections = Dapi.shared.bankConnections;
DAPIBankConnection *connection = connections[1];
NSLog(@"[DAPI] connection: %@",connection);
Get Bank Connections Parameters
A method for obtaining the connection parameters
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
let parameters = connection.getParameters()
print(parameters)
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
NSDictionary *parameters = [connection getParameters];
NSLog(@"[DAPI] connection parameters: %@",parameters);
Delete Bank Connections
To delete or delink the bank connection you can use delink
API, it will revoke the token and delete the bank connection from the SDK local cache.
let connections = Dapi.shared.bankConnections
let connection = connections.first(where: {$0.bankInfo.bankFullName == "Royal Bank of Canada" })
Dapi.shared.delink(bankConnection: connection) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiMetaData)
print(error.dapiErrorMessage)
}
}
DAPIBankConnection *connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
[Dapi.shared delinkWithBankConnection:connection
completion:^(DAPIResponseResult * _Nullable result, NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@", result);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
All set!That's it. You can use Bank Connection to allow user make payment initiation and data integration
Account Metadata
A method for obtaining information about the user's bank. Useful for knowing special capabilities or restrictions for the specific financial institution.
Parameters
Parameter | Description |
---|---|
bankConnection | A connection to the bank that will be used to call APIs upon. The connection will include details about the bank. Check How To Create Bank Connection |
Response
Check the Meta Data Response
Example
let connection = ... // selectedConnection or use Dapi.shared.connections to get cashed accounts
Dapi.shared.accountMetadata(bankConnection: connection) { results in
switch results {
case .success(let response):
print(response)
case .failure(let error):
print(error.dapiErrorMessage)
print(error.dapiMetaData)
}
}
DAPIBankConnection *connection = // selectedConnection or use Dapi.shared.connections to get cashed accounts;
[Dapi.shared accountMetadataWithBankConnection: connection
completion:^(DAPIAccountMetadataResponse * _Nullable response , NSError * _Nullable error) {
NSLog(@"[DAPI] Success: %@", response);
NSLog(@"[DAPI] Error: %@", error.dapiErrorMessage);
}];
Updated 14 days ago