Data API
getAccounts
Provides information about all the sub-accounts that the user has in their bank account.
Parameters
Method does not receive any parameter.
Example
await connection?.getAccounts()
.then(accounts => {
console.log('Accounts: ', accounts);
})
.catch(error => {
console.log('Dapi getAccounts() failed with error: ', error);
});
Response
Present Account Selection
Open Dapi's Accounts Screen to allow your user to select an account
await connection.presentAccountSelection();
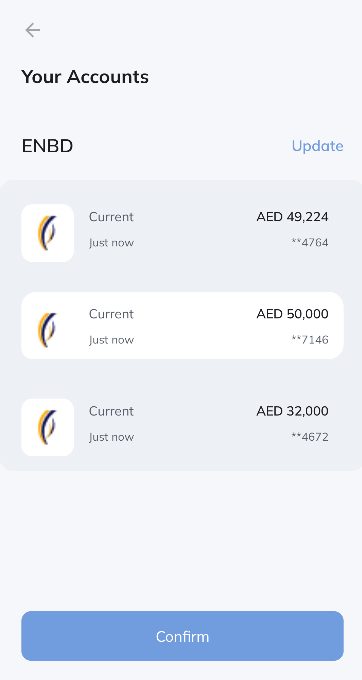
getIdentity
Get the identity information that has been confirmed by the bank.
These are the identity details that you will get. Not all banks provide all this data. So we will provide as much of it as possible.
Parameters
Method does not receive any parameter.
Example
await connection?.getIdentity()
.then(identity => {
console.log('Identity: ', identity);
})
.catch(error => {
console.log('Dapi getIdentity() failed with error: ', error);
});
Response
getCards
A method for obtaining and displaying credit cards of the user
Parameters
Method does not receive any parameter.
Example
await connection?.getCards()
.then(cards => {
console.log('Cards: ', cards);
})
.catch(error => {
console.log('Dapi getCards() failed with error: ', error);
});
Response
Account - getTransactions
A method for obtaining and displaying transactions created from users bank accounts. The list will not be filtered. In other words, this will display all the transactions performed by the user from the specified account (not filtered by app).
Parameters
Parameter | Description |
---|---|
account | Account from where the transaction was performed |
fromDate | Start date of transactions history range |
toDate | End date of transactions history range |
type | Enum to specify transactions type.DapiTransactionsType.enriched -> Each transaction object will have category and brandDetails properties.DapiTransactionsType.categorized -> Each transaction object will have category property.DapiTransactionsType.default -> category and brandDetails won't be included in the response. |
Example
let accountsResponse = await connection?.getAccounts();
await connection?.getTransactionsForAccount(
accountsResponse!.accounts[0],
new Date(startDateInMillis),
new Date(endDateInMillis),
DapiTransactionsType.default,
).then(transactions => {
console.log('Transactions: ', transactions);
})
.catch(error => {
console.log('Dapi getTransactionsForAccount() failed with error: ', error);
});
Response
Card - getTransactions
A method for obtaining and displaying transactions created from a card. The list will not be filtered. In other words, this will display all the transactions performed by the user from the specified account (not filtered by app).
Parameters
Parameter | Description |
---|---|
card | Credit card from where the transaction was performed |
fromDate | Start date of transactions history range |
toDate | End date of transactions history range |
type | Enum to specify transactions type.DapiTransactionsType.enriched -> Each transaction object will have category and brandDetails properties.DapiTransactionsType.categorized -> Each transaction object will have category property.DapiTransactionsType.default -> category and brandDetails won't be included in the response. |
Example
let cardResponse = await connection?.getCards();
await connection?.getTransactionsForCard(
cardResponse!.cards[0],
new Date(startDateInMillis),
new Date(endDateInMillis),
DapiTransactionsType.default,
).then(transactions => {
console.log('Transactions: ', transactions);
})
.catch(error => {
console.log('Dapi getTransactionsForCard() failed with error: ', error);
});
Response
Note
Date range of the transactions that can be retrieved varies for each bank. The range supported by the users bank is shown in the response parameter
transactionRange
ofGet Accounts Metadata
endpoint. If the date range you provide is bigger than thetransactionRange
you'll getINVALID_DATE_RANGE
error.To make sure you always send a valid date range you should check the transactionsRange.
Example:async function getTransactionsForNumberOfMonths(numberOfMonths: number) { let days = numberOfMonths * 30; let dayMillis = 24*60*60*1000 let response = await selectedConnection?.getAccountsMetadata() let transactionRange = response?.accountsMetadata.transactionRange let unit = transactionRange?.unit let value = transactionRange?.value if(unit == null || value == null) { //Can't check transactions range.. } else { var multiplier = 1; if (unit == "years") { multiplier = 365; } else if (unit == "months") { multiplier = 30; } else { multiplier = 1; } var transactionRangeValueInDays = value * multiplier; var fromDate = new Date(); if(transactionRangeValueInDays > days) { //numberOfMonths is within the transaction range fromDate = new Date(Date.now() - (days * dayMillis)) } else { //numberOfMonths is NOT within the transaction range, so we query only for the allowed range fromDate = new Date(Date.now() - (transactionRangeValueInDays * dayMillis)) } await selectedConnection?.getTransactionsForAccount( selectedConnection.accounts[0], fromDate, new Date(), DapiTransactionsType.default ); } }
Updated about 1 year ago